Understanding PHP Variable Scope
Scope is essentially about the visibility and accessibility of certain elements within a program, determining which parts can utilize them. In the realm of PHP, the concept of scope is closely linked with the operational structure of functions. To get a handle on scope, it’s crucial to first get acquainted with the fundamentals of functions. Think of a function as a set of instructions bundled together. A more detailed exploration of functions will be covered in our PHP functions tutorial.
If you’re just starting out and are not yet familiar with what functions are, this chapter might seem a bit overwhelming. Don’t let that deter you. Give it a preliminary read, then proceed to the other chapters. Once you’ve gone through the chapter on functions, it would be beneficial to revisit this section for a clearer understanding.
When delving into the realm of PHP data types, an understanding of variable scope in PHP becomes indispensable, as it greatly influences how these data types are accessed and manipulated within the code.
Types of Variable Scope in PHP
Variable scope in PHP can be divided into three distinct categories:
- Global Scope;
- Local Scope;
- Static Scope.
Understanding Global Scope in PHP
In PHP, variables that are declared in the main body of the script, outside any function, are endowed with a global scope. However, this designation of global scope doesn’t automatically permit their use inside functions. These globally scoped variables remain inaccessible within function boundaries unless specifically referenced. To gain a clearer insight into this concept, it’s helpful to look at the illustrative example given below:
<?php
$number = 10; // global scope
echo $number; // outputs 10
function num() {
echo $number; // $number is undefined here
}
Exploring Local Scope in PHP
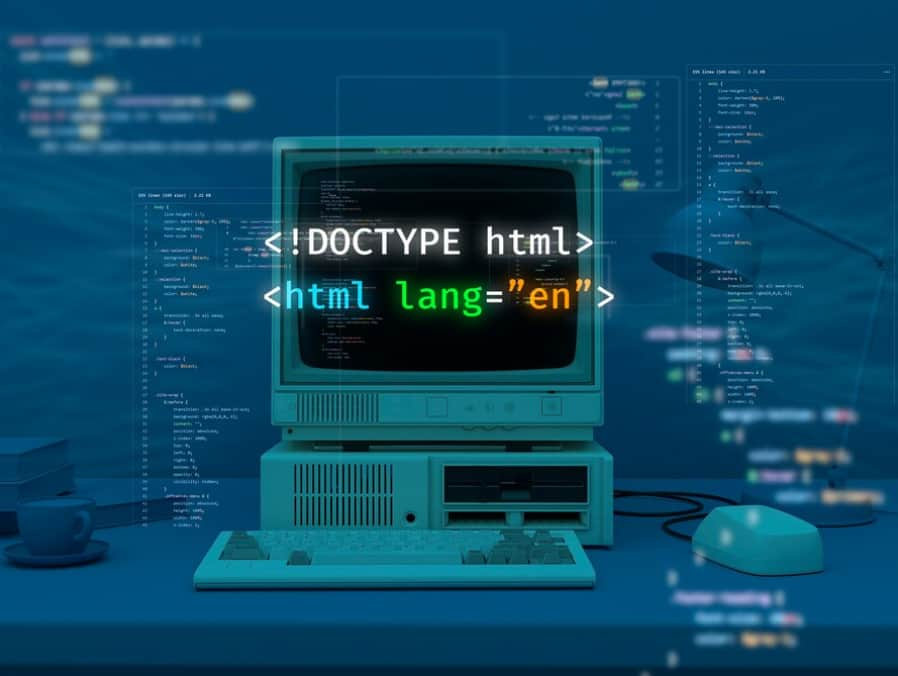
Elements defined with a local scope are confined to the boundaries of a specific function. Unique to the function where they are established, these elements remain accessible solely within that particular function’s scope. This unique trait enables the simultaneous existence of multiple elements with identical names across various functions, avoiding any overlap or conflict. When the function hosting a locally scoped element concludes its execution, the element is no longer in existence, and the memory allocated to it is subsequently freed up.
Here’s an example demonstrating local scope:
<?php
function hello() {
$txt = 'Hello World'; // local scope
echo $txt; // $txt can be used here
}
hello();
// $txt cannot be used outside
PHP Global Variables in Local Scope
There are two methods by which global variables can be accessed within a function:
- Utilizing the global keyword;
- Employing the $GLOBALS array.
Using the PHP Global Keyword
In PHP, the global keyword serves a pivotal role in enabling access to globally defined variables from within a function’s scope. To make use of this feature, it’s important to first declare the global keyword, followed by listing the needed identifiers, separated by commas. This method ensures that globally scoped identifiers are integrated into the localized environment of a function. It broadens the scope, allowing for access and manipulation of these identifiers within the function’s framework.
Refer to the example below:
<?php
$x = 'Hyvor';
$y = 'Developer';
function websiteName() {
global $x, $y;
echo $x, $y;
}
websiteName(); // outputs HyvorDeveloper
// Removing global $x, $y; from the code will lead to an error as $x and $y variables are not defined within the function websiteName().
Utilizing PHP $GLOBALS Array
PHP stores all global variables in a pre-defined $GLOBALS array. This array’s key comprises the variable’s name, whilst its value contains the variable’s value. Consider the example below:
<?php
$x = 'Hyvor';
$y = 'Developer';
function websiteName() {
echo $GLOBALS['x'], $GLOBALS['y'];
}
websiteName(); // outputs HyvorDeveloper
Note: An array is another data type in PHP, which we will delve deeper into in our PHP Arrays tutorial.
PHP Static Scope: A Detailed Look
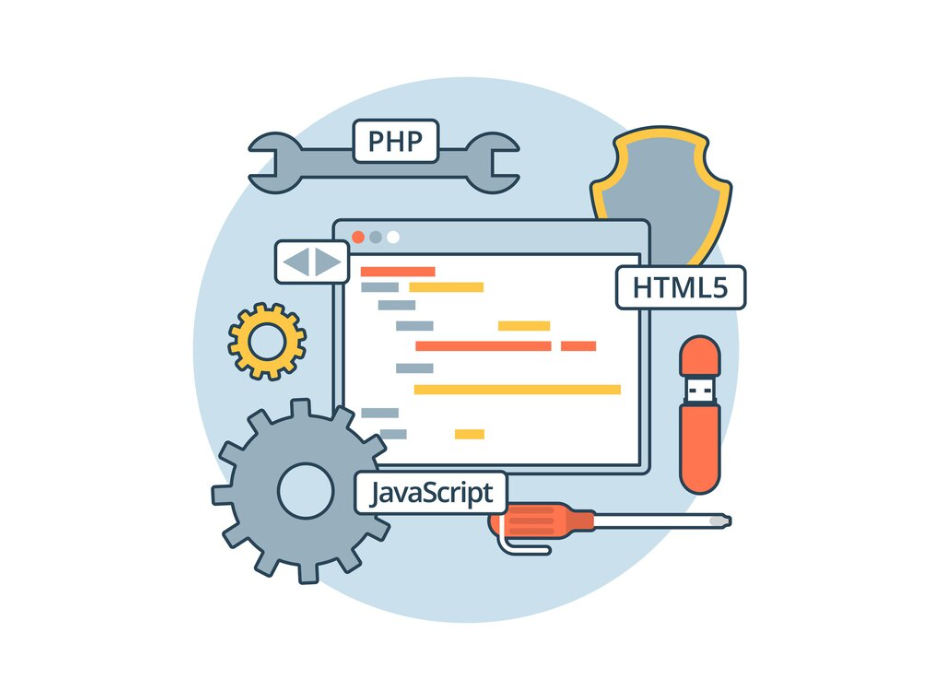
Previously, we explored how variables with local scope are removed once the function they’re in concludes. However, there are occasions where retaining these elements is necessary.
To achieve this, one should employ the static keyword during their declaration.
Working with PHP Static Variables
To make a variable static, add the static keyword while declaring the variable. Here’s an example that demonstrates this:
<?php
function test() {
static $number = 0; // declare static variable
echo $number . '<br>'; // echo number with line break
$number = $number + 5; // add five to $number
}
test(); // 0
test(); // 5
test(); // 10
Upon each execution of the function, the $number variable retains the value from the last assignment.
Conclusion
Understanding variable scope in PHP is crucial for effective and error-free coding. PHP offers various types of variable scopes, including global, local, static, and superglobal, each with its own purpose and visibility rules. Global variables can be accessed from anywhere within a script, but they should be used sparingly to avoid potential conflicts and maintain code modularity. Local variables are confined to their respective functions or blocks, ensuring encapsulation and preventing unintended side effects. Static variables retain their values across function calls, while superglobal variables provide access to data that can be shared across different parts of a script. A solid grasp of variable scope in PHP is essential for writing maintainable, efficient, and organized code in web development and beyond.