In the world of programming, a “string” holds a prominent place. Often underestimated, they play a critical role in the storage and manipulation of data. So, what exactly is a string? In the simplest terms possible, a string is a finite sequence of characters. These characters can include anything: alphabets, numerals, symbols, punctuation marks, and even spaces. But it’s not just about storing these characters. It’s also about how these strings are used and manipulated in a programming language.
PHP, a widely-used open-source scripting language known for web development and general-purpose programming, has unique rules for handling string values. Learning PHP strings is crucial for anyone looking to master PHP.
How to Declare Strings in PHP?
PHP offers programmers multiple ways to declare strings. Depending on the context or requirement, you can choose the most suitable approach. Here’s a closer look at each method:
- Single Quoted Strings: This approach is the simplest way to define a string. Simply enclose any set of characters using single quotes;
- Double Quoted Strings: This method is similar to its single-quoted counterpart but it interprets more escape sequences and variables;
- Heredoc Syntax: If you’re dealing with large blocks of text, heredoc syntax can be a lifesaver. It offers the flexibility of double-quoted strings but with added readability;
- Nowdoc Syntax: Nowdoc is the single-quoted equivalent of heredoc. It doesn’t parse escape sequences or variables and is suitable for embedding larger text blocks that need to remain exactly as they are.
Choosing the right method has significant implications. For instance, single-quoted strings consume less processing power than their double-quoted counterparts while Heredoc and Nowdoc syntaxes improve readability for large text blocks but may complicate simple string usages.
Single Quoted Strings
One of the simplest ways to declare a string in PHP is by using single quotes (’ ‘).
Consider this example:
$string = 'This is my first string';
Our variable $string holds the string ‘This is my first string’ enclosed in single quotes (’ ‘).
But what happens when our text needs to include single quotes too? That’s where escaping comes in.
To include a single quote in a single quoted string, precede it with a backslash (), like so:
$string = 'This is the Hyvor\'s PHP Tutorial';
Now, you could be faced with another conundrum: what if you need to include the sequence ” in the string? Once again, escaping is the solution. Use two backslashes (\) to represent a single backslash () and follow the same rule for the single quote.
Example:
$string = 'Backslash and Quote: \\\'';
Escaping is an essential concept in PHP strings. Escape sequences help eliminate confusion and grant special meaning to certain characters when included within a string. Getting the hang of escaping takes a bit of practice, but once it’s understood, coding in PHP becomes much easier.
Double Quoted Strings in PHP: A Detailed Guide
Apart from single quotes, PHP also allows you to declare strings using double quotes (” “). Using double quotes instead of single quotes enhances the functionality of strings in your PHP scripts.
In PHP, certain characters and character combinations have special meanings when used within a double quoted string. These are known as escape sequences. An escape sequence starts with a backslash () and is used to insert special characters into the string without breaking the code.
Escape sequences bear different meanings within the context of a double quoted string, as detailed in the table below:
Escape Sequence | Significance |
---|---|
\n | Insert a newline in the text at this point. |
\r | Implements a carriage return. |
\t | Inserts a tab space. |
\ | Inserts a backslash. |
$ | Inserts a dollar symbol. |
“ | Inserts a double quote in the string. |
Making use of escape sequences can transform the way you manipulate strings in PHP.
Let’s take a look at an example of PHP code using double quoted strings with escape sequences:
echo "Hello, World!";
echo "<br>";
echo "\"Hello, World!\"";
echo "\n\tHello, World\n";
In this code snippet, we’ve used the double quotes to encapsulate different strings. The escape sequences used include \” for including double quotes within the string, and \n\t for a new line and a tab space, respectively.
A Detailed Guide to Variables in Double Quoted Strings in PHP
Strings in PHP, especially those declared with double quotes, have an interesting feature: variable parsing. When you place a variable within a double-quoted string, PHP automatically identifies it and replaces it with its assigned value within the string. This functionality makes string manipulation more dynamic and adaptable to changing data.
Let’s see this in action with a simple PHP code snippet:
$name = "Hyvor";
echo "My name is $name";
Here, the variable $name within the string is automatically replaced by its value, resulting in the output: My name is Hyvor.
Handling PHP Variables: Advanced Techniques
Now let’s explore a scenario that requires a slightly more sophisticated understanding of variable handling within double-quoted strings. Suppose you need to add a character or a string directly after a variable without a space intervening.
Consider this example:
$name = "Hyvor";
echo "My name is $name";
In our $fruit example, we want to pluralize our fruit name by adding an ‘s’. But if we just added ‘s’ after the variable without anything else, PHP might consider it to be a different variable ($fruits, which we have not declared). The solution? Use curly braces ({}) to wrap around the variable name, helping PHP distinguish where the variable name ends and where the attached string (‘s’ in our case) begins.
Unveiling the Differences Between Single and Double Quoted Strings in PHP
Distinguishing between single and double quoted strings in PHP, though seems simple on the surface, can spiral into an intricate territory that intertwines functionality, escape sequences, and variable parsing.
To simplify this, here’s a rundown of the key differences:
Attributes | Single Quoted Strings | Double Quoted Strings |
---|---|---|
Literal Interpretation | Yes, displays exactly as typed | No, interprets enclosed elements |
Variable Parsing | No, variables are not recognized | Yes, variables are replaced by their values |
Escape Sequences | Limited, only supports ‘ and \ | Comprehensive, supports a wide range of escape sequences |
To see how these differences play out in real PHP coding, consider the following examples:
// Both single and double quotes do their job
echo "Hello <br>";
echo 'Hello <br>';
// Escape sequences in single quotes are not interpreted
echo "\"World\" <br>"; // Outputs "World"
echo '\"World\" <br>'; // Outputs \"World\"
// Variables in single quotes are not parsed
$greeting = "Hello";
echo "$greeting World <br>"; // Outputs Hello World
echo '$greeting World <br>'; // Outputs $greeting World
As seen in these examples, double quotes have the upper hand in terms of flexibility. They interpret escape sequences and parse variables within the string. However, this extra functionality also means they might require slightly more processing power than single quoted strings.
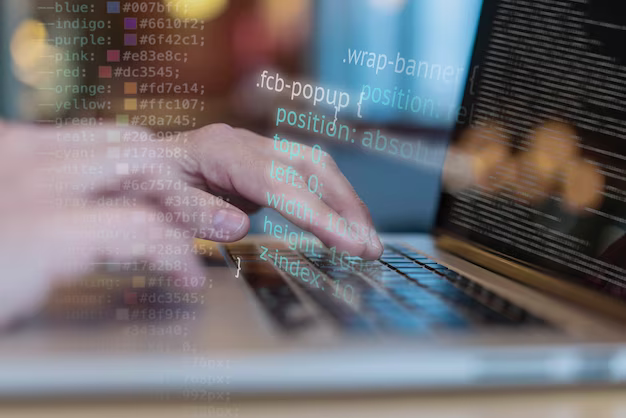
Exploring the Power of Doc Syntax in PHP
Doc Syntax in PHP provides a unique way of declaring strings that looks different from the conventional single or double quoted methods. It comes in two flavors, each resembling the behavior of single and double quoted strings:
- Nowdoc syntax: Mirrors the behavior of single quoted strings;
- Heredoc syntax: Replicates the behavior of double quoted strings.
Nowdoc Syntax
Nowdoc syntax is the PHP equivalent of single quoted strings in the PHP Doc Syntax. Just like single quoted strings, Nowdoc does not interpret escape sequences or variables within the string.
To declare a string using Nowdoc syntax, start with <<<, followed by an identifier enclosed in single quotes. The identifier should be a valid PHP label, starting with a non-digit character or underscore, and only containing alpha-numeric characters and underscores. The string should start on a new line after the identifier, and the identifier must be on a new line at the end of the string, followed by a semi-colon (;).
Here’s an example of a string declared using Nowdoc syntax:
$message = <<<'MSG'
Hello,
Welcome to PHP Tutorials!
MSG;
echo $message;
This code would output the text exactly as it is written.
Heredoc Syntax
Heredoc syntax resembles the behavior of double quoted strings. It interprets escape sequences and parses variables within the string.
Declaring a string using Heredoc syntax is similar to the Nowdoc method, except the identifier is not enclosed in quotes.
Here’s an example of a string declared using Heredoc syntax:
$name = "Hyvor";
$message = <<<MSG
Hello $name,
Welcome to PHP Tutorials!
MSG;
echo $message;
This code would output the text with the variable $name replaced with its assigned value.
How to Insert Image in Database Using AJAX in PHP
To enrich your PHP projects, consider incorporating image handling via AJAX. This entails utilizing asynchronous JavaScript and XML (AJAX) to interact with a PHP script that manages the storage and retrieval of images in a database. By doing so, you can create dynamic web applications that allow users to upload, display, and manipulate images seamlessly.
Here are the essential steps to achieve this:
- HTML Form: Create an HTML form that includes an input field for image uploads. Use the <input type=”file”> element to allow users to select image files for uploading;
- AJAX Request: Write JavaScript code to capture the selected image file and send it to a PHP script using AJAX. The PHP script will handle the image insertion into the database;
- PHP Script: Develop a PHP script that receives the image file, processes it, and stores it in the database. You may need to use PHP libraries like PDO or MySQLi to interact with the database;
- Database Setup: Ensure your database schema includes an appropriate table to store image data, with a column specifically designed for storing binary image data;
- Response Handling: Implement a response mechanism in your AJAX code to display feedback to the user, confirming whether the image insertion was successful or not.
By merging this image insertion capability with your proficiency in PHP string manipulation, you can create dynamic web applications that handle both textual and visual content seamlessly. This synergy between PHP’s string handling and database integration empowers developers to craft versatile and engaging web experiences.
Wrapping Up
In conclusion, understanding the intricacies of string manipulation in PHP is paramount for any programmer looking to excel in this versatile scripting language. We have explored various methods of declaring strings, the significance of escape sequences, and the dynamic nature of variable parsing within double-quoted strings. Additionally, we have uncovered the unique power of Doc Syntax, both Nowdoc and Heredoc, in PHP. Armed with this knowledge, programmers can harness the full potential of PHP strings, making their code more efficient, readable, and adaptable to a wide range of applications. Mastery of these concepts not only elevates one’s programming skills but also ensures the proper handling of string values in PHP, a crucial element in web development and general-purpose programming.