Type casting is a critical concept in any programming language. And PHP is not an exception to this. In the realm of PHP, it refers to the process of converting a variable from one data type to another – like from an integer to a float.
Understanding the Concept of Type Casting in PHP
In PHP, you will notice a distinct feature. Unlike certain other languages such as C++, you aren’t required to specify the variable’s data type during its declaration. PHP automatically assigns to it. This is a fundamental aspect of PHP that sets it apart from strictly-typed languages.
However, while the PHP parser automatically sets the data type, you might find yourself needing to change it. This could be due to a requirement in your code or to manipulate the variable in a manner that its original data type may not support. In these scenarios, the variable’s data type can be converted, either automatically or manually. This process of converting the data type is what we call “Type Casting.”
Delving Deeper: The Two Types of Casting
Type casting in PHP can be categorized into two.
- Implicit Casting (Automatic);
- Explicit Casting (Manual).
Decoding Implicit Casting in PHP
Implicit casting, also known as automatic type conversion, is a clever feature of PHP. It takes the burden of specifying the data type off your shoulders and handles it according to your code. In simpler words, PHP decides the result of an operation based on the values that you are using in your calculations.
For instance, when you divide one integer by another, the outcome could be an integer or a float. However, the decision about the data type of the result isn’t made by a developer but is handled automatically by PHP itself.
PHP Implicit Casting in Action
To understand this better, let’s visualize this with an example:
<?php
$numberOne = 2;
$numberTwo = 4;
var_dump($numberOne / $numberTwo); // Result: 0.5, which is a float
var_dump($numberTwo / $numberOne); // Result: 2, which is an integer
?>
In the code above, PHP automatically decides the data type of the result. When $numberOne is divided by $numberTwo, since the result is a decimal number, PHP automatically casts the result as a float. Conversely, when $numberTwo is divided by $numberOne, PHP casts the result as an integer because it’s a whole number. Thus, PHP automatically determines the necessary data type according to the needs of the operation.
Ultimately, implicit casting allows PHP to automatically handle the conversion of data types based on the operation. It saves programmers time and effort from specifying the data type for every operation. However, it is important to understand how PHP decides on the data type so you can anticipate the outcome and debug issues efficiently.
Exploring Explicit Casting in PHP
Explicit Casting, also known as manual type conversion, provides more control over how data types are manipulated in PHP. As the name suggests, explicit casting is when the programmer manually changes the data type of a variable. For instance, it allows you to convert a floating-point number to an integer. In this case, the decimal part of the float gets truncated, and only the integer part is kept.
PHP Explicit Casting – Converting Float to Integer
Let’s consider this with an example:
<?php
$valueA = 5.35;
$valueB = (int) $valueA; // valueA is cast to an integer
var_dump($valueB);
?>
In the above code, $valueA is a float. But when we use (int) before $valueA, it converts $valueA into an integer by dropping the decimal part. The output will be int(5). Therefore, $valueB becomes an integer. This is explicit casting in PHP where we, the programmers, have explicitly indicated the type conversion.
Explicit Casting – a Look at Available Casts
The data type to which the variable needs to be converted must be mentioned in parentheses before the variable.
Here is a broad classification of casts available in PHP:
- (int) or (integer): Converts the variable to an integer;
- (float) or (double) or (real): Changes the variable into a float;
- (bool) or (boolean): Transforms the variable into a boolean;
- (string): Converts the variable to a string;
- (array): Changes the variable into an array;
- (object): Transforms the variable to an object.
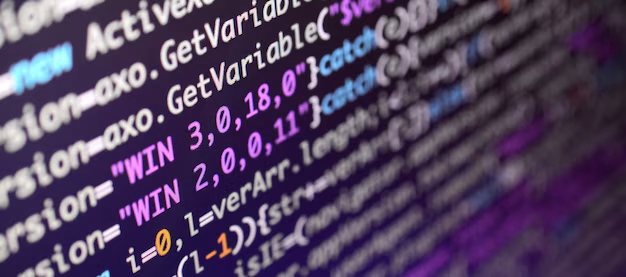
Explicit Casting Examples in PHP
Explicit Casting empowers PHP developers by giving them control over the data type of a variable. This ability to switch between data types is very helpful in specific programming scenarios. Let’s dive deeper into explicit casting with some examples:
1. Casting a Float to an Integer
In PHP, a float can be cast to an integer using both (int) and (integer). During this conversion, PHP discards the decimal part of the float. Therefore, it’s crucial to ensure this loss of data doesn’t unintentionally impact other aspects of the program.
<?php
$numA = 5.35;
$numB = (int) $numA; // cast $numA to integer
var_dump($numB); // Result: int(5)
?>
In the above code, $numA is a float that is then explicitly cast as an integer. The decimal portion is removed, and $numB is returned as an integer 5.
2. Casting a String to an Integer
Casting strings to integers is a practical application of explicit casting, especially when handling numeric data retrieved from forms.
<?php
$stringNum = '25';
$intNum = (integer) $stringNum; // cast $stringNum to int
var_dump($intNum); // Result: int(25)
?>
In this case, $stringNum is a string that presents a numeric value. When (integer) is used, $stringNum is converted to an integer, and the result is 25.
3. Casting a Phrase to an Integer
Interestingly, in PHP, a phrase that begins with a number can also be cast to an integer. The output will be the initial number of the string.
<?php
$phrase = '10 Animals';
$countOfAnimals = (int) $phrase;
echo $countOfAnimals; // Output: 10
?>
In this example, $phrase starts with the number ‘10.’ When we cast $phrase to an integer, PHP extracts the initial number and assigns it to $countOfAnimals.
Unveiling the Synchrony of PHP Class Constants and Type Casting
In the multifaceted landscape of PHP, class constants reign as stalwart pillars of stability. Much like type casting, they offer a framework of rigidity within the otherwise dynamic realm of programming. PHP class constants facilitate the association of immutable values with classes, ensuring that these values remain constant throughout the class’s lifecycle. While they may appear distinct from the concept of type casting, both elements underscore PHP’s versatility and depth. Just as type casting allows for the flexible manipulation of data types, class constants provide a steadfast reference point within the codebase, enhancing code readability and maintainability.
Conclusion
Understanding type casting can dramatically improve a developer’s PHP coding proficiency. Whether it’s manipulating data types automatically with implicit casting or controlling conversions manually using explicit casting, both skills offer essential benefits. By exploring these concepts and their applications, we can interact with data in more dynamic ways, enrich our PHP development skills, and craft more intricate and powerful code.