A constructor, in the context of PHP, is defined as a type of public method with the specific name __construct. This unique method is automatically invoked when an instance of a class is created.
PHP Constructors: Laying the Foundation
A constructor, as part of PHP’s object-oriented programming architecture, serves as a public method specifically referred to as __construct.
In essence, it is a predefined, automatic method invoked during an instance creation of a class. Its primary role is to set initial values for class properties.
Consider the following elementary example:
class Sample {
public function __construct() {
// Code encapsulated in the constructor function
echo "Greetings, Universe!";
}
}
In this instance, the PHP __construct() method is triggered immediately upon the creation of a class object.
$sampleObject = new Sample(); // Outputs "Greetings, Universe!"
Now, let’s delve into a comprehensive example utilizing a constructor. We’ll structure a __construct() method with two parameters, $alias and $hue. These would be passed during object instantiation.
PHP Constructor Example: A Deeper Dive
<?php
class Residence {
public $alias;
public $hue;
public function __construct($alias, $hue) {
$this -> alias = $alias;
$this -> hue = $hue;
}
public function revealDetails() {
echo "The {$this -> hue} residence belongs to {$this -> alias}";
}
}
$redResidence = new Residence("Mike's Abode", "red");
$redResidence -> revealDetails();
Here, the Residence class contains a __construct() method with two arguments — $alias and $hue. These object properties attain their values when an object is instantiated. The revealDetails() method is then employed to echo the residence’s owner and color.
Constructing Without a Constructor: Handy Tips
Interestingly, even without a constructor or a constructor without parameters, PHP allows class object creation sans parentheses.
$residence = new Residence;
However, if a constructor with parameters exists, remember to assign values to those parameters during object creation.
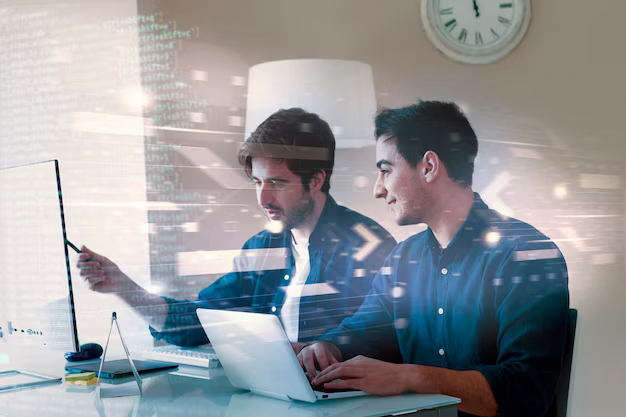
PHP Destructors: The Unsung Heroes
Conversely, another critical facet of PHP’s object-oriented structure is a destructor, presented as __destruct method. While the constructor is automatically called when an object is created, the destructor is invoked when an object is discarded, or the script execution is halted or terminated.
Consider the following, detailed PHP destructor example:
<?php
class Building {
public $designation;
public $shade;
public function __construct($designation, $shade) {
$this -> designation = $designation;
$this -> shade = $shade;
}
public function __destruct() {
echo "The {$this -> shade}-colored structure belongs to {$this -> designation}";
}
}
$whiteBuilding = new Building("David's Studio", "white");
In the code snippet above, note that the __destruct() method is called post script execution. It essentially wraps up the object’s lifetime and can be utilized for cleanup activities such as closing database connections or releasing resources.
Important Points to Consider While Using Destructors
- The __destruct() procedure is summoned when the present PHP script has been entirely executed, arriving at the last line, or while terminating it using exit() or die()functions;
- Unlike __construct, the __destruct() method does not permit any parameters.
Exploring the Dark Web: A Tangential Yet Pertinent Inquiry
In the vast universe of digital exploration, there exists an enigmatic realm known as the “dark web.” While seemingly unrelated to PHP constructors and destructors, the dark web is, in its own right, a cryptic domain. Often shrouded in secrecy, it serves as a parallel dimension where anonymity and clandestine activities thrive. However, it’s imperative to note that the dark web’s intricacies lie beyond the scope of this discourse, which primarily centers on the complexities of PHP programming.
Conclusion
In the realm of PHP programming, constructors and destructors are not merely methods but powerful management tools. They dictate the object lifecycle, ensure efficient resource utilization, and provide valuable insights during debugging. As such, their understanding and effective use are paramount for any PHP programmer. As you continue your journey deeper into PHP, let these built-in methods be your guide, shaping your path towards becoming an astute and proficient PHP coder.