A function in programming serves as a procedural or routine block, containing a set of statements that encapsulate specific functionality. Functions are designed to remain inert until explicitly invoked, allowing them to be called repeatedly whenever necessary, making them a versatile and reusable tool in code development.
Understanding default arguments in PHP is essential when dealing with .htaccess files for web server configuration. If you’re intrigued by this topic, you may also find interest in exploring advanced PHP concepts and further web server optimization techniques.
PHP’s Extensive Range of Built-In Functions
PHP boasts an extensive array of built-in functions, each meticulously designed to execute specific tasks. In previous discussions, we’ve covered a few of these functions:
- echo() – Used for outputting strings;
- define() – Employed to establish constants;
- var_dump() – Utilized for displaying variable data.
Throughout this tutorial, we’ll delve into other PHP built-in functions, expanding your knowledge step by step.
Creating Custom Functions in PHP
In PHP, you can create your own functions for specific tasks. Defining a function involves using the “function” keyword, followed by the function’s name and its arguments.
Here’s the basic syntax for declaring a user-defined function:
function functionName(arg1, arg2, ....) {
code to be executed
}
Guidelines for Naming User-Defined Functions in PHP
Naming your user-defined functions in PHP follows similar rules to variable naming, with one key distinction: functions do not start with the “$” sign.
Here are some essential guidelines for naming user-defined functions:
- A function name should commence with a letter or an underscore;
- It cannot begin with a number;
- After the initial character, you can use letters, numbers, and underscores in the function name;
- Function names are case-insensitive in PHP, meaning that “boom()” and “Boom()” both refer to the same function.
A helpful tip: Always choose function names that provide a clear description of their intended purpose, enhancing the readability and maintainability of your code.
Creating Your First User-Defined Function in PHP
Now, let’s embark on creating our inaugural user-defined function in PHP;
- To begin, we declare our function named “greet()” using the function syntax;
- The enclosed code within the curly braces ({}) represents the function’s executable code. This code is executed whenever we invoke the function;
- Finally, we initiate the function by calling it using its name, followed by parentheses: greet();
Keep in mind that in PHP, parentheses are essential for invoking a function.
Below is an example of a PHP user-defined function:
<?php
function greet() {
$hour = date('G'); // get the hour in 24-hour format
if ($hour < 12) {
echo 'Good Morning';
} else if ($hour < 17) {
echo 'Good Afternoon';
} else {
echo 'Good Night';
}
}
greet(); // calling the function
Function Parameters in PHP
Functions in PHP can yield different outcomes by utilizing arguments as inputs.
Function arguments represent the values passed into a function, effectively functioning as variables within the function’s scope.
To define function arguments:
- Place them within the parentheses immediately following the function name;
- Multiple arguments can be accommodated, separated by commas;
- Argument names must adhere to the same rules as variable names since they essentially act as variables within the function.
Let’s illustrate this concept with an example:
- We start by declaring a function called “myName” with a single argument, “$name.”;
- Subsequently, we invoke the function multiple times, each time providing a different value within the parentheses of the function call. This value gets assigned to the “$name” variable when the function executes.
Here is a demonstration of PHP function arguments in practice:
<?php
function myName($name) {
echo 'My name is ' . $name;
echo '<br>'; // line break
}
myName('Joe');
myName('Adam');
myName('David');
Functions can accept multiple arguments.
Example of PHP functions with multiple arguments:
<?php
function myDetails($name, $age, $country) {
echo "
My name is $name <br>
My age is $age <br>
My country is $country <br><br>
";
}
myDetails('Joe', 22, 'USA');
myDetails('Adam', 25, 'United Kingdom');
myDetails('David', 30, 'France');
PHP Function Arguments – Passing by Reference
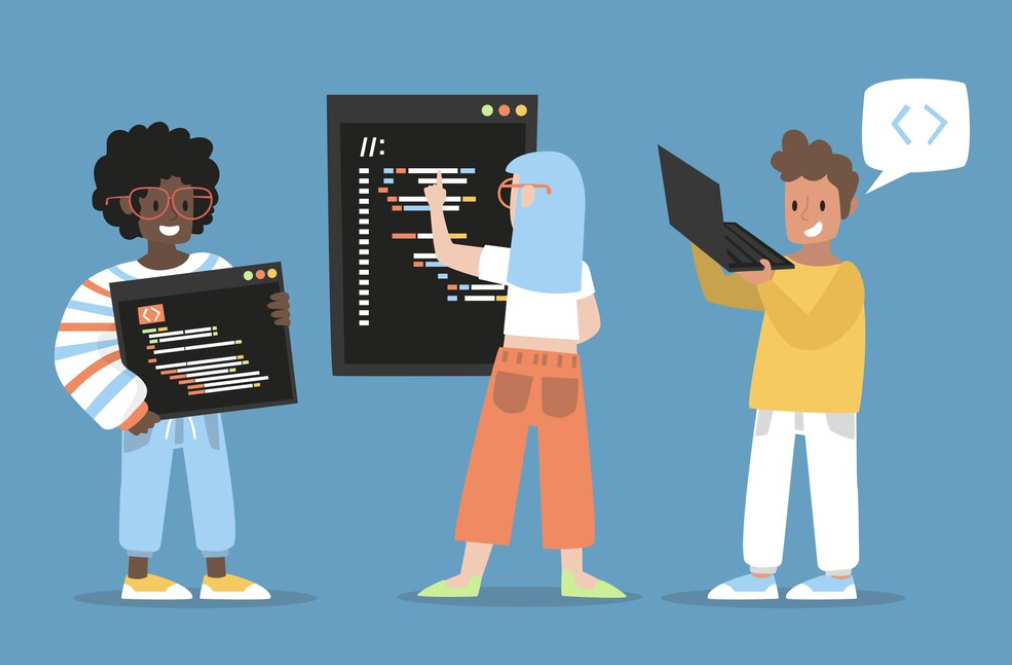
By default, PHP functions pass arguments by value. To better understand this concept, consider the following example:
PHP passing arguments by value example:
<?php
function changeName($name) {
$name .= ' Developer';
echo 'Inside the function: ' . $name . '<br>'; // outputs "Hyvor Developer"
}
$rootName = 'Hyvor';
changeName($rootName);
echo 'Outside the function: ' . $rootName; // it is stil 'Hyvor'
In the above example:
- The $rootName variable is initially set to “Hyvor.”;
- It is then passed as the $name argument to the changeName() function;
- Inside the function, “Hyvor” is modified to “Hyvor Developer.”;
- However, after the function execution, the global variable $rootName retains the value “Hyvor.”
If you want to modify a variable within a function and have those changes reflected outside the function, you can pass it by reference. To achieve this, simply prepend an ‘&’ sign to the argument name in the function definition.
PHP passing arguments by reference example:
<?php
function changeName(&$name) {
$name .= ' Developer';
echo 'Inside the function: ' . $name . '<br>'; // outputs "Hyvor Developer"
}
$rootName = 'Hyvor';
changeName($rootName);
echo 'Outside the function: ' . $rootName; // now it's 'Hyvor Developer'
In this revised example, the $rootName variable is modified within the function when passed by reference, and the change persists outside the function, resulting in “Hyvor Developer” when echoed outside the function.
PHP Function Arguments with Default Values
You can assign default values to function arguments in PHP by using the basic assignment operator (=) in the function definition. When calling the function, if an argument is not provided, the default value specified in the definition will be used.
PHP dunctions with default argument values example:
<?php
function printNumber($number = 10) {
echo "The number is: $number <br>";
}
printNumber(2);
printNumber(25);
printNumber(); // will print 10, the default value
printNumber(500);
PHP Function Arguments – Type Declaration
In PHP, you can utilize type declarations, also known as type hinting, to specify the expected data type for each function argument. If the provided argument does not match the specified data type, PHP will generate an error. To implement type declarations, you simply add the desired data type before each argument in the function definition.
PHP functions with type declaration in arguments example:
<?php
function myDetails(string $name, int $age, string $country) {
echo "
My name is $name <br>
My age is $age <br>
My country is $country <br><br>
";
}
myDetails('Joe', 22, 'USA');
myDetails('Adam', 25, 'United Kingdom');
myDetails('David', 30, 'France');
# myDetails('John', 'N/A', 'Australia'); this will cause an error
Valid Data Types for Type Declaration in PHP
Type | Description | Minimum PHP Version |
---|---|---|
array | The argument must be an array | 5.1.0 |
callable | The argument must be a callable (function or method) | 5.4.0 |
int | The argument must be an integer | 7.0.0 |
float | The argument must be a float | 7.0.0 |
bool | The argument must be a boolean | 7.0.0 |
string | The argument must be a string | 7.0.0 |
PHP Functions – Return Statements
In PHP functions, return statements serve two primary purposes:
- To return a value from a function;
- To terminate the execution of a function when a specific condition becomes true.
PHP Functions – Returning Values
Let’s explore the first use case, which involves returning values from functions.
PHP functions – returning values example:
<?php
function sum($num1, $num2) {
return $num1 + $num2;
}
echo '5 + 5 = ' . sum(5,5) . '<br>';
echo '4 + 3 = ' . sum(4,3) . '<br>';
echo '8 + 1 = ' . sum(8,1) . '<br>';
PHP Functions – Type Declaration for Returned Values
In PHP, you have the capability to specify the data type of the value returned by a function. This feature helps enforce strict typing and clarifies the expected data type for the return value. The available data types for return value type declaration are the same as those used for argument type declaration.
Consider the following example:
<?php
function sum($num1, $num2) {
return $num1 + $num2;
}
echo '5 + 5 = ' . sum(5,5) . '<br>';
echo '4 + 3 = ' . sum(4,3) . '<br>';
echo '8 + 1 = ' . sum(8,1) . '<br>';
In the above code, the sum() function is declared with a return type declaration of float. This means that the function is expected to return a value of type float. If the result is not already a float, PHP will attempt to cast it to float when returning. For example, if the result is an integer, it will be automatically cast to float and then returned. However, if you attempt to return a value of a different data type, such as a string, PHP will throw an error.
Variable Functions in PHP
In PHP, when you append parentheses to a variable that contains a string, PHP checks if there is a function with a matching name and proceeds to execute it. This concept is known as variable functions.
PHP Variable Functions – An Illustrative Example
In PHP, you can employ variable functions to dynamically call functions based on the value of a variable. This powerful feature allows you to determine which function to execute at runtime.
PHP variable functions example:
<?php
function sum($num1, $num2): float {
return $num1 + $num2;
}
var_dump(sum(5, 2)); // Outputs a float value
?>
PHP Anonymous Functions
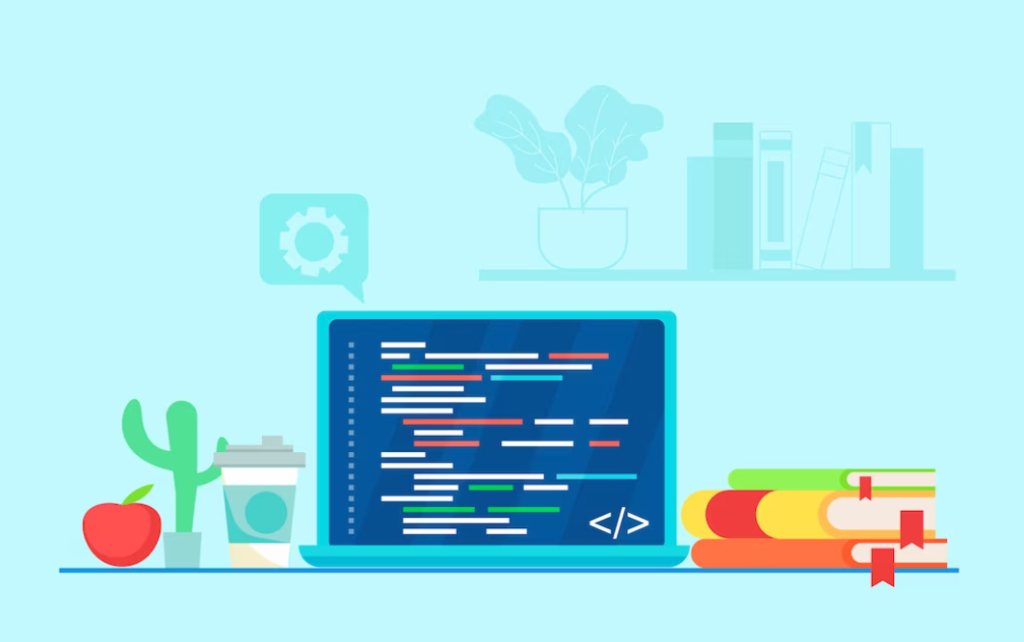
Anonymous functions, also known as closures, are functions that lack a formal name. They prove to be immensely valuable when you need to pass callback arguments to other functions.
Example: PHP Anonymous Functions
<?php
function callFunc($callback) {
$x = rand();
$callback($x);
}
// Calling callFunc() with an anonymous function as its argument
callFunc(function($number) {
echo $number;
});
?>
In the provided example, we pass an anonymous function as the first parameter to the callFunc() function. This anonymous function takes a single parameter $number and echoes its value. It demonstrates how anonymous functions can be used as callback mechanisms within PHP.
While anonymous functions may initially appear complex, we will explore their applications further in subsequent chapters with additional examples to make their usage more comprehensible.
Conclusion
Understanding the structure and role of PHP functions is pivotal for any aspiring PHP developer. These small sets of instructions can be called upon to perform specific tasks, greatly improving code efficiency and maintainability. The ability to declare your own custom functions also allows a higher level of flexibility in your code. Passing variables by reference and the use of default argument values can greatly enhance your programming prowess and allows for more complex and flexible codes. The in-depth knowledge about variable functions and anonymous functions further enhances your versatility as a developer. Henceforth, mastering these concepts will prove to be a tremendous boost when writing PHP scripts.