Constants are critical in computer programming. They are unchanging values that define key aspects of a program’s functionality. Unlike variables, constants remain the same throughout a program, providing a reliable and predictable element in software development. Their use ranges from setting configuration values to defining fixed points in algorithms, making them integral to efficient and clear coding.
Defining Constants: A Programmer’s Steadfast Ally
In the world of programming, constants are defined as values that do not change. Once a constant is set, it maintains that value throughout the program. This immutability contrasts with the variable nature of variables, which can change over time. Constants are essential for error-free programming, offering a fixed point of reference in the otherwise fluid environment of software development. They simplify the code, making it more readable and maintainable.
Types of Constants: A Spectrum of Invariability
Constants can be categorized into two primary types:
- Literal Constants: These are the most basic form of constants. They represent fixed values directly inserted into the code and do not change. For example, numeric values like 42 or string values like “Hello, World!” are literal constants. They are straightforward and often used for values that are self-explanatory within the context they are used;
- Declared Constants: These constants are explicitly declared in the code and are assigned a name for ease of reference and readability. For instance, in a C++ program, you might declare const int MAX_USERS = 100;. This declaration ensures that MAX_USERS remains at 100 throughout the program, enhancing both the code’s clarity and maintainability.
Type | Example | Usage |
---|---|---|
Literal Constants | 50, “Welcome” | Directly used in code for fixed values |
Declared Constants | const float PI = 3.14 | Named constants for easy reference |
The Role of Constants in Different Programming Languages
Each programming language has its unique approach to defining and using constants. For instance:
- JavaScript: Constants are declared using the const keyword, as in const PI = 3.14;
- Python: Python does not have built-in support for constants. However, it is a convention to use all uppercase letters for naming constants, like MAX_SIZE = 100;.
Constants in Action: Real-World Applications
Constants find their application in various programming scenarios:
- Configuration Values: They are used to set application-wide settings, such as screen dimensions (SCREEN_WIDTH = 1024;) or default fonts (DEFAULT_FONT = “Arial”;);
- Algorithmic Anchors: In algorithms, constants act as fixed points. For example, in recursive functions, a constant can define the base case which stops the recursion.
The Significance of Constants in Code Optimization
Using constants can significantly enhance the performance and readability of the code. They help in:
- Reducing Processing Overhead: Constants eliminate the need for recalculating values, thereby saving processing time;
- Enhancing Code Clarity: By providing descriptive names to values, constants make code more understandable and maintainable.
Constants and Coding Standards: A Harmonious Blend
Adhering to coding standards and conventions is crucial when using constants. This includes:
- Consistent Naming Conventions: Using a consistent naming scheme across the codebase enhances readability and maintainability;
- Effective Scope Management: Managing the scope of constants effectively prevents conflicts and confusion, especially in large codebases.
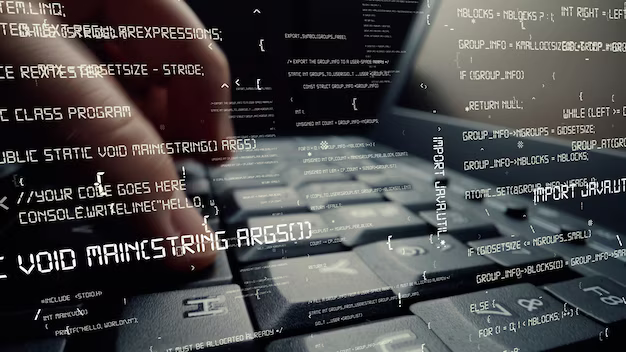
PHP Forms Validation with Constants: Enhancing Security and Maintainability
In PHP web development, incorporating constants into form validation is an effective strategy to enhance both security and efficiency. By defining key validation parameters as constants, the process becomes more streamlined and consistent.
Example: Streamlining Validation with Constants
In a user registration form, you might have to validate an email and password. Using constants for criteria simplifies this:
define("MAX_EMAIL_LENGTH", 255);
define("MIN_PASSWORD_LENGTH", 8);
$email = $_POST['email'];
$password = $_POST['password'];
if (strlen($email) > MAX_EMAIL_LENGTH) {
// Error handling for email length
}
if (strlen($password) < MIN_PASSWORD_LENGTH) {
// Error handling for password length
}
Benefits of Constants in PHP Form Validation
- Consistency: Constants ensure uniform standards across the application;
- Maintainability: Changing a single constant updates the validation rule application-wide;
- Readability: Clear, descriptive constant names make the code more understandable.
Conclusion
Constants are more than just fixed values; they are the backbone of programming that provides stability and predictability in a dynamic environment. Their effective usage is key to writing code that is not only efficient and reliable but also easy to understand and maintain. As we continue to advance in the world of programming, the role of constants remains ever significant, anchoring logic and functionality in the seas of change.