Regular Expressions, often abbreviated as regex, offer a specialized syntax tailored for parsing and managing text. What makes them advantageous? The answer is straightforward: They enable rapid and efficient pattern detection within text sequences. Capable of executing numerous functions such as searching and substituting text, validating user input, among others, they significantly boost your coding productivity.
After learning about PHP Regular Expressions, we’ll next tackle PHP Forms Security to keep user data safe.
Defining Regular Expressions (Regex)
Regular expressions—also known as Regex or RegExp—allow you to match patterns within data strings. They can range from simple characters to complex, intricate patterns, all governed by specific rules. This guide will walk you through the creation of regular expressions and demonstrate how to apply them within PHP functions.
Regular Expressions in PHP
PHP integrates well with regex, thanks to its support for the widely used syntax known as PCRE (Perl Compatible Regular Expressions). The available PCRE functions in PHP are prefixed with “preg_,” providing a robust set of tools for various string operations.
Sample PHP Regex Code: Replace Operation Example
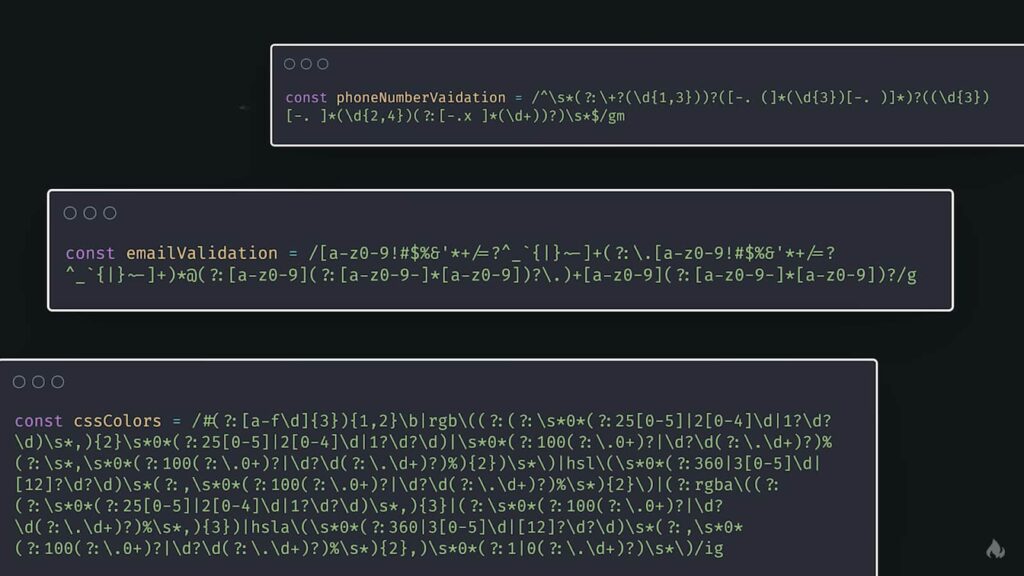
Consider this PHP code, which utilizes the preg_replace function:
<?php
$str = 'Hello World';
$regex = '/\s/';
echo preg_replace($regex, '', $str);
?>
In this snippet, the first space in “Hello World” is targeted and removed, resulting in “HelloWorld”. But how does it work? Here’s a breakdown:
- The preg_replace() function searches for a specific pattern within a string and replaces it with another string;
- The $regex variable is responsible for defining the search pattern;
- The / characters enclosing $regex are delimiters, marking the start and end of the regex;
- \s is a regex expression matching any whitespace characters;
- Finally, the match is replaced with ‘’, effectively removing the whitespace.
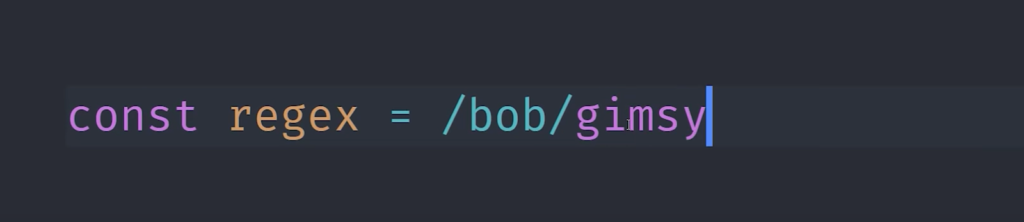
PHP Regex Functions
PHP comes equipped with an extensive range of native functions dedicated to regular expressions. Functions such as preg_match(), preg_match_all(), and preg_split() cater to diverse scenarios. Grasping how these functions work can greatly enhance your capabilities in PHP text manipulation.
Getting to grips with the principles that dictate regex patterns can greatly refine your proficiency in crafting accurate search criteria. A host of special metacharacters, quantifiers, and character sets are at your disposal to assemble intricate patterns.
Conclusion
PHP’s regular expression functionality allows for advanced pattern matching and text-processing tasks. Whether you are validating text inputs, performing search and replace operations, or even splitting strings, regex is a vital tool in your PHP arsenal. The secret lies in mastering the pattern rules and the arsenal of PHP functions at your disposal. Remember, practice is key to becoming proficient with regex!