In Object-Oriented Programming (OOP) with PHP, namespaces function as a way to group several classes under the same label. This prevents potential naming conflicts and offers a higher degree of organization within your development environment.
If you found the discussion on PHP namespaces informative, you may also like our article on the mysqli – prepare, where we dive into practical aspects of database management in PHP.
Understanding Variable Scope and Namespacing in PHP
Concept 1: Variable Scope in PHP
Imagine working with a PHP script where a variable named $title exists in the global scope. Now, within a specific function, you declare another variable with the exact same name, $title, and modify its value. Despite these changes within the function, the global $title variable remains unaffected. This illustrates the concept of variable scope. Similar principles apply to classes, where namespacing can be used to define and manage their scope.
Concept 2: Avoiding Name Collisions in PHP Libraries
Consider you’re developing an open-source PHP library for sending emails, which includes a class named Email. If another developer, who already has a class called Email, uses your library, they’ll encounter a name collision. To resolve this, either the class names must be changed, or a more elegant solution can be employed: utilizing namespaces to differentiate between the two.
Concept 3: Simplified Explanation of Namespacing
Just as two individuals can share the name “Jack” but be distinguished by their surnames, classes in PHP can have identical names but be differentiated using namespaces. This approach allows for clear identification and prevents conflicts in larger, more complex codebases.
Functionality and Organization through Namespaces
Namespaces are crucial in preventing name collisions and enhancing code organization. This chapter will guide you on how to effectively use namespaces for these purposes.
Importance of Namespaces in PHP
In PHP, having two classes with the same name within the same scope is not allowed. For instance, if you need separate classes for managing blog users and app users, you might name them Blog_User and App_User. However, managing such prefixed names can become cumbersome. Namespaces offer a more streamlined and organized approach, providing greater control over class identification and management.
Namespaces in Relation to File Structure
The concept of namespaces in PHP is analogous to file structures in operating systems. Consider namespaces as folders and classes as files within these folders:
- In a `/lib/math` folder, you might have various files like `numbers.php`, `calculus.php`, etc., with no duplicate file names;
- Accessing `numbers.php` from within `calculus.php` is straightforward and direct;
- Similarly, a `/lib/physics` folder could contain `mechanics.php`, `electromagnetism.php`, and even its own `numbers.php`, distinct from the one in /lib/math;
- Within the physics folder, referencing `numbers.php` directly will point to `/lib/physics/numbers.php`;
- If you need to access `numbers.php` from the math folder while in the `physics` folder, the path `/lib/math/numbers.php` must be used;
- This file structure metaphor illustrates how namespaces function, allowing for organized and clear referencing of classes.
Understanding the Global Namespace
In PHP, any class, function, or constant declared without a specific namespace is considered part of the global namespace. This concept is akin to storing files directly on a computer’s hard drive, outside any specific folder. Understanding this helps in differentiating between items in the global namespace and those in specific, user-defined namespaces.
Declaring Namespaces in PHP
To declare a namespace in PHP, the namespace keyword is used, followed by the desired namespace name. For example:
<?php
namespace MyApp;
The chosen name for the namespace should comply with the PHP identifier rules. Importantly, the namespace declaration must be placed at the beginning of the PHP file, with the only exception being the declare keyword.
Namespaces can encompass various PHP code elements, but primarily impact classes, interfaces, constants, and functions. While it’s technically feasible to declare multiple namespaces in a single file, this practice is generally discouraged for clarity and maintainability.
Creating and Using Namespaced Classes
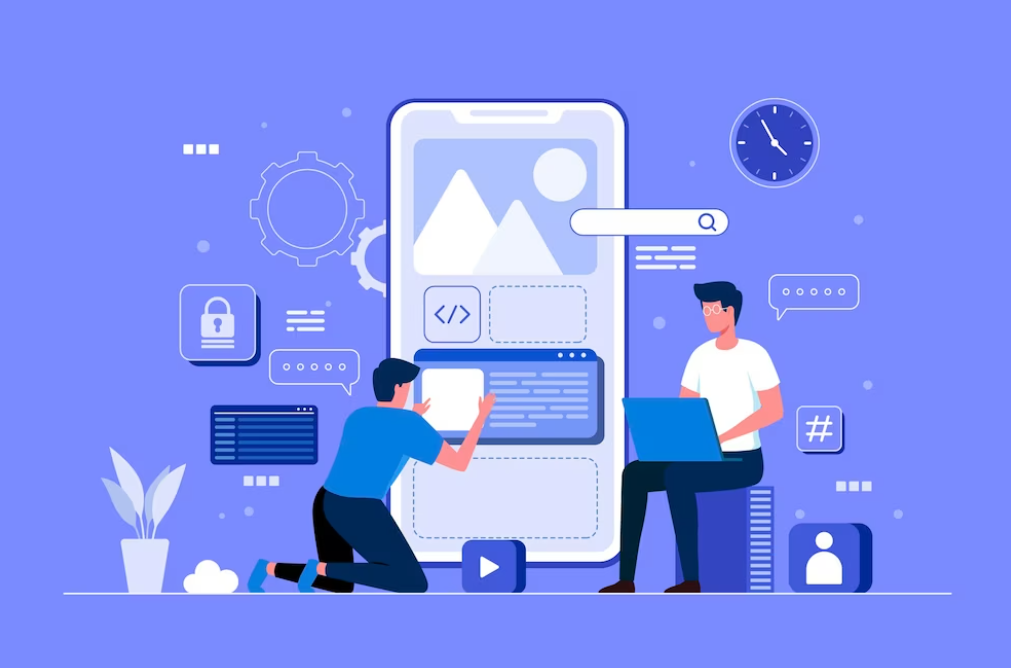
An important principle in this tutorial is PHP’s relative nature to the current namespace. By adding a namespace declaration at the top of a PHP file, all subsequently defined classes, functions, and constants inherently become part of that namespace.
Consider a file named Math.php with the following code:
Math.php:
<?php
namespace Math;
function add($a, $b) {
return $a + $b;
}
const PI = 3.14;
class Geometry {
static function getCircleArea($radius) {
return PI * $radius ** 2;
}
}
- Namespace Declaration: Begin by declaring the namespace, which ensures that all following classes, interfaces, constants, and functions are part of this namespace;
- Function Definition: Next, define a standard function within this namespace;
- Class Constant: Following that, introduce a constant specific to the class;
- Class Declaration: Then, declare a class, which also falls under the defined namespace;
- Accessing Namespace Components: Lastly, create a new file named usage.php. In this file, demonstrate accessing the namespace’s components like functions, constants, and classes, as defined earlier.
usage.php:
<?php
// includes the Math.php file
// It's like you had all the code of Math.php written here
include_once 'Math.php';
echo Math\add(2,3); // 5
echo Math\PI; // 3.14
echo Math\Geometry::getCircleArea(10); // 314.15
The \ character is employed to descend one level within namespaces.
Hierarchical Namespaces in Programming
In programming, particularly within operating systems, the concept of folders containing other folders is a common organizational structure. This concept extends to namespaces in programming, where namespaces can encompass other namespaces, referred to as sub-namespaces.
Consider this PHP example:
<?php
namespace Math\Geometry;
// ... your geometry classes
This snippet indicates that we’re delving into the world of Object-Oriented Programming (OOP) within this tutorial, where the emphasis will be solely on classes within namespaces, moving away from functions and constants.
Optimal Namespace Organization in Object-Oriented PHP
In a forthcoming chapter, we’ll explore the intricacies of Object-Oriented programming in PHP. For now, our focus is on effectively structuring namespaces, a crucial aspect to grasp.
Here are some guidelines for namespace organization:
- Namespace and Directory Alignment: It’s advisable to align your namespaces with the local directory structure on your computer. For instance, if your project resides in the /var/www directory, you might organize your PHP classes within the /var/www/src folder;
- Namespace-Directory Correspondence: Each namespace should have a corresponding directory bearing the same name (including matching letter cases). For example, a directory for the Math namespace would be /var/www/src/Math;
- Class File Organization:
- Each class should be encapsulated in its own PHP file (.php extension);
- One PHP class file should contain only one class definition;
- For example, the Math\Addition class would be located at /var/www/src/Math/Addition.php.
- Structuring Sub-Namespaces: Sub-namespaces should have dedicated directories for their classes. For instance, classes belonging to the Math\Geometry sub-namespace should be in /var/www/src/Math/Geometry.
It’s important to note that these are recommendations rather than rigid rules. They are designed to promote clarity and organization in your code, but you have the flexibility to adapt them as per your project’s needs.
Illustration – Math Namespace in Action
To gain a better grasp of the concept, let’s dive into an example. In this demonstration, we will construct a namespace called “Math” and populate it with sub-namespaces and classes. Here’s a breakdown of the structure:
- Main Namespace: Math;
- Sub-Namespace: Math\Geometry (Designed for handling geometric operations);
- Classes:
- Math\Constants (Intended for storing commonly used constants);
- Math\Geometry\Circle (Dedicated to circle-related computations);
- This is the finalized structure of our project, providing a practical insight into organizing namespaces and classes.
/src /Math /Geometry Circle.php
Constants.php
Math/Constants.php
<?php
namespace Math;
class Constants {
const PI = 3.14159;
}
Math/Geometry/Circle.php
<?php
namespace Math\Geometry;
class Circle {
public $radius;
public function __construct($radius) {
$this -> radius = $radius;
}
public function getDiameter() {
return $this -> radius * 2;
}
public function getArea() {
// (pi)(r^2)
return \Math\Constants::PI * $this -> radius ** 2;
}
public function getCircumference() {
// 2(pi)(r)
return 2 * \Math\Constants::PI * $this -> radius;
}
}
In Circle.php, we’ve utilized \Math\Constants::PI to reference the constant located in the Constants.php file. This approach is necessary because PHP interprets namespaces as relative to the current namespace. When a namespace begins with a backslash (), the name is resolved with respect to the global namespace.
If we were to employ Constants::PI in Circle.php, it would point to \Math\Geometry\Constants::PI.
Similarly, using Math\Constants::PI (without the initial backslash) in Circle.php would lead to \Math\Geometry\Math\Constants::PI. This underscores that class names are contingent on the current namespace, and the backslash () is employed to return to the global namespace. It’s essential to note that PHP does not support relative namespaces (e.g., ../Constants::PI).
Lastly, let’s incorporate our classes into index.php, which should reside in the root folder of your project.
<?php
include_once 'src/Math/Constants.php';
include_once 'src/Math/Geometry/Circle.php';
$circle = new Math\Geometry\Circle(10);
echo $circle -> getDiameter(); // 20
echo $circle -> getArea(); // 314.159
echo $circle -> getCircumference(); // 62.8318
Initially, we imported the class files and subsequently employed the Math\Geometry\Circle class alongside its associated methods. It’s crucial to highlight that index.php exists in the global namespace, as it is not situated within any specific namespace.
The “use” Statement for Class Import within Namespaces
The “use” keyword serves as a powerful tool for importing classes into the current scope within namespaces.
By including the following code snippet in your index.php file, the Math\Geometry\Circle class will be readily imported into the current scope, allowing you to utilize its functionalities seamlessly.
<?php
use Math\Geometry\Circle;
$circle = new Circle(10); // as Circle class is now in this namespace
Importing Both Namespaces and Classes is Possible
<?php
use Math\Geometry; // importing an namespace
use Math\Geometry\Circle; // importing a class
Employing Aliases for Imports
Aliases, also known as nicknames, can be employed when importing classes in two distinct scenarios:
- When you have an existing class with the same name as the imported class.
- When you desire a more convenient or simplified name for the imported class.
To create aliases, you make use of the “as” keyword during the import process.
<?php
use Math\Geometry\Circle as Circ;
$circle = new Circ(10);
Modifying the Previous Example with Import Statements
Let’s make adjustments to our index.php file from the previous example by incorporating “use” statements to import the class.
<?php
// ... import statements
use Math\Geometry\Circle;
$circle = new Circle(10);
// ... calling methods
In the absence of an “as” keyword, the imported class can be referenced simply as “Circle.” However, we can also import the Circle class with an alias.
<?php
use Math\Geometry\Circle as C;
$circle = new C(10);
But, it’s important to note that using overly concise aliases like “C” is not considered a good practice. Always opt for descriptive and meaningful names.
Here’s a task for you: Utilize the “use” statement as demonstrated in Circle.php and substitute “\Math\Constants::PI” with a more concise alias.
Structuring Code for Enhanced Organization with Namespaces
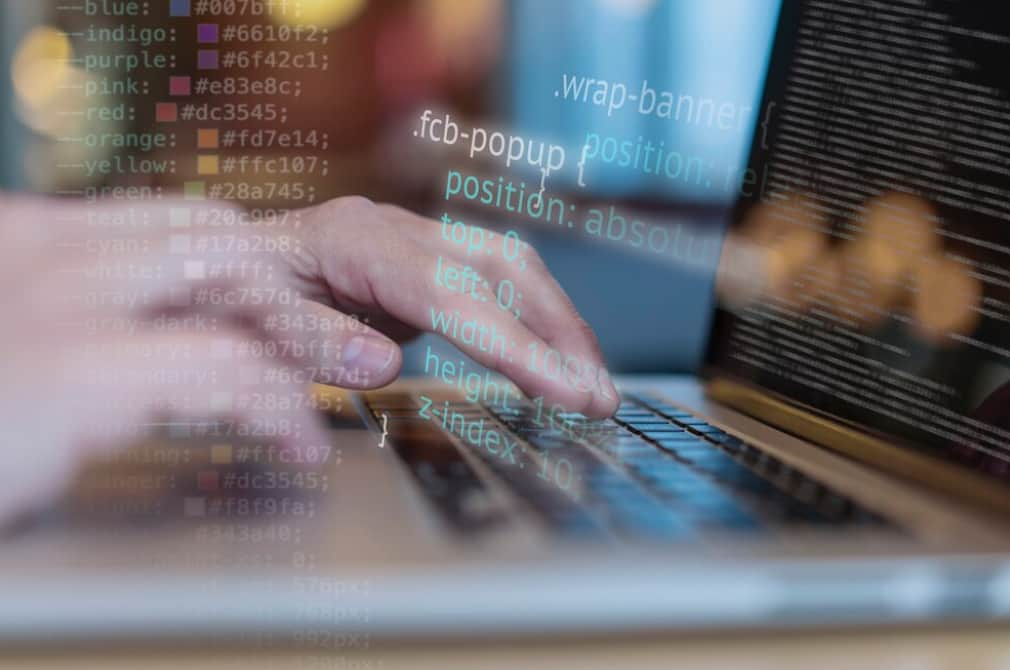
Namespaces serve as a fundamental tool for effectively organizing code. Let’s explore this concept within the context of developing an open-source library.
When crafting your library, one primary objective is to avoid naming conflicts. To achieve this, consider the following practices:
Practice | Description |
---|---|
Namespace All Classes | Incorporate all classes within a designated namespace, typically named after yourself or your library. This ensures a distinct and organized namespace for your codebase. |
Utilize Subnamespaces | Group classes with similar functionalities into subnamespaces. This hierarchical approach maintains a logical structure within your codebase, making it easier to navigate and manage. |
Adhere to Folder Structure | Implement the folder structure discussed earlier to align with your namespace hierarchy. This approach ensures that your code is organized cohesively, both in terms of namespaces and directories. |
Conclusion
This tutorial covered the concept of namespaces, their purpose, and methods for their implementation. Utilizing namespaces effectively leads to a more organized and manageable project structure. While there is flexibility in their usage, adhering to common practices recommended by many developers is beneficial.