Have you ever witnessed the marvel of a real-time search in action? Simply enter a query into the search bar at the pinnacle of your web browser, and behold as it swiftly furnishes you with a cascade of suggestions while you type. This ingenious method is commonly referred to as Live Search.
To truly grasp the intricacies of this concept, immerse yourself in the role of a live search user. Now, let’s delve into the intricacies of implementing it programmatically using the dynamic duo of AJAX and PHP.
Creating the AJAX-Enhanced Search Feature
Enhancing your website with an AJAX-powered search feature can significantly improve user experience by delivering real-time results as users type into the search box. In this section, we’ll guide you through creating the foundation for this feature using HTML and JavaScript.
1. Setting up index.html
Your index.html is the starting point for this project. It will contain the search box and the result container. Here’s how you can structure it:
<!DOCTYPE html>
<html>
<head>
<title>AJAX Search</title>
</head>
<body>
<input type="text" name="username" id="textbox" placeholder="Enter your search query" />
<div id="result"></div>
<script type="text/javascript">
// JavaScript code goes here
</script>
</body>
</html>
Added a placeholder text to guide users. Consider adding CSS to style your elements for a visually appealing interface.
2. Declaring JavaScript Variables
To interact with your HTML elements, declare JavaScript variables as shown below:
var textBox = document.getElementById('textbox');
var resultContainer = document.getElementById('result');
var ajax = null;
var loadedUsers = 0;
- textBox and resultContainer store references to the DOM elements selected by their respective id attributes;
- ajax will hold an instance of XMLHttpRequest() for making asynchronous requests;
- loadedUsers keeps track of the number of users loaded and displayed. This is useful when implementing infinite scrolling or loading more suggestions.
3. Adding onkeyup Event
To trigger the search as the user types, add the onkeyup event to the search box:
textBox.onkeyup = function() {
var val = this.value;
val = val.trim(); // Remove leading and trailing spaces
if (val !== "") {
searchForData(val);
} else {
clearResult();
}
};
- We use the trim() method to eliminate leading and trailing whitespace;
- When the input is not empty, it calls the searchForData() function, otherwise, it clears the result container;
- The use of onkeyup ensures that the search is triggered after the user releases a key, providing a more responsive experience compared to onkeydown.
4. Creating searchForData() and clearResult() Functions
In the next step, you’ll create the searchForData() and clearResult() functions. These functions will handle fetching and displaying search results as well as clearing the result container when needed.
Creating an AJAX Request with the searchForData() Function
In this section, we’ll delve into the intricacies of the searchForData() function, a crucial component of your web application. This function is responsible for handling AJAX requests, which are pivotal for dynamically loading data from the server. Let’s break it down step by step, making it more comprehensive and informative:
function searchForData(value, isLoadMoreMode) {
// Abort previous AJAX requests if they exist
if (ajax && typeof ajax.abort === 'function') {
ajax.abort();
}
// Clear the result container if we're not in load more mode
if (isLoadMoreMode !== true) {
clearResult();
}
// Create a new XMLHttpRequest object
ajax = new XMLHttpRequest();
// Set up an event listener to handle the response
ajax.onreadystatechange = function () {
if (this.readyState === 4 && this.status === 200) {
try {
var json = JSON.parse(this.responseText);
} catch (e) {
noUsers();
return;
}
if (json.length === 0) {
if (isLoadMoreMode) {
alert('No more results to load');
} else {
noUsers();
}
} else {
showUsers(json);
}
}
}
// Construct the AJAX request
var requestURL = 'search.php?username=' + value + '&startFrom=' + loadedUsers;
ajax.open('GET', requestURL, true);
ajax.send();
}
Key Points:
- The searchForData() function accepts two parameters: value and isLoadMoreMode;
- value represents the user’s input string;
- If isLoadMoreMode is set to true, the function won’t clear the result container, allowing for additional results to be appended;
- Any previous AJAX requests are aborted to avoid interference with the current request;
- The result container is cleared if we’re not in load more mode;
- A new XMLHttpRequest object is created to facilitate the AJAX request;
- An event listener is set up to handle the response from the server;
- The constructed AJAX request URL includes the user’s input string and the starting point for matching results;
- Upon successful response, the JSON data is parsed, and appropriate actions are taken based on the data length.
Constructing the AJAX Request URL
Now, let’s dive deeper into how the AJAX request URL is constructed, providing more insights into this critical process:
var requestURL = 'search.php?username=' + value + '&startFrom=' + loadedUsers;
The URL is built dynamically, incorporating two essential parameters:
- username: Represents the user’s input string, allowing the server to search for matching results;
- startFrom: Specifies where the server should start fetching results. For instance, if the function has already loaded 6 user suggestions, the startFrom value ensures that the server skips the initial 6 results and sends the subsequent ones.
Handling and Displaying Data with showUsers()
Now, let’s explore the showUsers() function in more detail. This function plays a pivotal role in converting raw data into a user-friendly display:
function searchForData(value, isLoadMoreMode) {
// Abort previous AJAX requests if they exist
if (ajax && typeof ajax.abort === 'function') {
ajax.abort();
}
// Clear the result container if we're not in load more mode
if (isLoadMoreMode !== true) {
clearResult();
}
// Create a new XMLHttpRequest object
ajax = new XMLHttpRequest();
// Set up an event listener to handle the response
ajax.onreadystatechange = function () {
if (this.readyState === 4 && this.status === 200) {
try {
var json = JSON.parse(this.responseText);
} catch (e) {
noUsers();
return;
}
if (json.length === 0) {
if (isLoadMoreMode) {
alert('No more results to load');
} else {
noUsers();
}
} else {
showUsers(json);
}
}
}
// Construct the AJAX request
var requestURL = 'search.php?username=' + value + '&startFrom=' + loadedUsers;
ajax.open('GET', requestURL, true);
ajax.send();
}
Key Points:
- The showUsers() function is responsible for converting the raw data into a user-friendly format;
- It defines a nested createRow() function to create individual user display elements;
- Each user’s name, picture, and a clickable feature to show their description are included;
- The function loops through the data and generates a user display for each entry;
- A ‘Load More’ button is appended to allow users to retrieve additional results;
- An event listener is set up for the ‘Load More’ button, triggering the searchForData() function in load more mode when clicked;
- The count of loaded users is updated accordingly.
Clearing Results and Handling Empty Data
In this section, we’ll explore two crucial auxiliary functions: clearResult() and noUsers(). These functions play vital roles in managing the user interface when no results are available or when the results need to be cleared:
function clearResult() {
// Clear the result container
resultContainer.innerHTML = "";
// Reset the count of loaded users
loadedUsers = 0;
}
function noUsers() {
// Display a "No Users" message in the result container
resultContainer.innerHTML = "No Users";
}
Key Points:
- clearResult() function clears the result container by setting its HTML content to an empty string;
- Additionally, it resets the count of loaded users to zero;
- noUsers() function displays a “No Users” message in the result container, informing users when no matching results are found.
Establishing a Connection Between PHP and MySQL
When integrating PHP with a MySQL database, it’s crucial to understand the process of establishing a secure and efficient connection. This connection is the foundation for any dynamic web application, allowing PHP scripts to interact with database content.
Key Steps for Establishing a PHP-MySQL Connection:
- Database Credentials: Securely store your database username, password, host, and database name;
- Connection Script: Utilize PHP’s mysqli_connect() or PDO (PHP Data Objects) to create a connection script. This script should handle any connection errors gracefully, displaying user-friendly error messages without revealing sensitive information;
- Database Selection: Once connected, select the appropriate database to interact with, if not already specified in the PDO connection string.
Crafting a MySQL Table for User Details
A well-structured MySQL table is essential for storing and retrieving user information efficiently. The table should be designed to hold relevant user details, ensuring data integrity and optimal performance.
Steps to Create a MySQL Table:
- Define Table Structure: Decide on the necessary columns, such as id, name, picture, and description. Each column should have an appropriate data type and constraints;
- Use of SQL Commands: Utilize the CREATE TABLE SQL statement to define the table structure. For instance, an AUTO_INCREMENT primary key for the id column ensures unique identifiers for each record.
Example SQL Command:
CREATE TABLE users (
id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(60) NOT NULL,
picture VARCHAR(1000) NOT NULL,
description VARCHAR(1000) NOT NULL
)
Data Normalization: Consider normalizing the data to reduce redundancy and improve database efficiency.
Populating the Table with Data
Inserting data into the MySQL table is a fundamental operation. This step involves adding records to the table, which can then be used for various operations like search, update, and deletion.
Guidelines for Inserting Data:
- SQL Insert Statement: Use the INSERT INTO statement to add data. Remember, the id field will auto-increment, so there’s no need to specify it;
- Sample Data Insertion: Start by inserting a few records to test the table’s functionality. Ensure that the data types match the column specifications.
Example Insertion Command:
INSERT INTO users (name, picture, description) VALUES ('Example Name', 'Example URL', 'Example Description')
Data Validation: Before inserting, validate the data to maintain the integrity of the database.
Crafting the Live Search Handler
In this comprehensive guide, we will delve into the intricate process of creating a live search handler using PHP and MySQL. This invaluable tool will enable you to perform real-time searches and provide suggestions to users in JSON format. We’ll walk you through each crucial step, from establishing a database connection to executing a MySQL query with prepared statements. Buckle up for a journey into the world of dynamic search functionality!
Step 1: Establishing a MYSQL Connection
Before we can embark on our live search adventure, we need to establish a secure connection between PHP and MySQL. The following code snippet simplifies this task for you. However, remember to replace the placeholders ($host, $user, $password, and $database) with your actual database credentials.
<?php
$host = 'localhost';
$user = 'root';
$password = '';
$database = 'dev_testing';
$mysqli = new mysqli($host, $user, $password, $database);
$mysqli = new mysqli($host, $user, $password, $database);
Recommendation:
- Always store your database credentials securely and consider using environment variables for added security;
- Use a try-catch block to handle database connection errors gracefully.
Step 2: Validating AJAX Variables
A cardinal rule in web development is to never trust user inputs. Before utilizing any $_POST or $_GET variables, it is imperative to perform proper validation. In our case, we are dealing with $_GET variables, username and startFrom. Here’s how to sanitize and validate them:
$username = $_GET['username'];
$startFrom = $_GET['startFrom'];
$username = trim(htmlspecialchars($username));
$startFrom = filter_var($startFrom, FILTER_VALIDATE_INT);
Insight:
- trim() removes leading and trailing white spaces from the username;
- htmlspecialchars() prevents potential cross-site scripting (XSS) attacks;
- filter_var() with FILTER_VALIDATE_INT ensures that startFrom is a valid integer.
Step 3: Executing the MySQL Query with Prepared Statements
To protect your application from SQL injection attacks, always employ prepared statements when injecting dynamic values into your queries. Let’s dissect the core of our live search functionality:
// Make username search-friendly
$like = '%' . strtolower($username) . '%';
// Open a new mysqli prepared statement
$statement = $mysqli -> prepare('
SELECT name, picture, description
FROM users
WHERE lower(name) LIKE ?
ORDER BY INSTR(name, ?), name
LIMIT 6 OFFSET ?'
);
if (
$statement &&
$statement -> bind_param('ssi', $like, $username, $startFrom) &&
$statement -> execute() &&
$statement -> store_result() &&
$statement -> bind_result($name, $picture, $description)
) {
$resultArray = [];
while ($statement -> fetch()) {
$resultArray[] = [
'name' => $name,
'picture' => $picture,
'description' => $description
];
}
echo json_encode($resultArray);
exit();
}
Expanded Explanation:
- The $like variable ensures a case-insensitive search by converting both the input username and database names to lowercase;
- We create a prepared statement using $mysqli->prepare() to protect against SQL injection;
- The query selects name, picture, and description from the users table based on the user’s input;
- The ORDER BY clause sorts results based on their similarity to the input username, providing more relevant suggestions first;
- We check for successful execution and binding of parameters before proceeding;
- The result is fetched into an associative array, and the final JSON response is sent to the client.
Also, dive into the fascinating realm of PHP Object-Oriented Programming (PHP OOP) and unlock endless coding possibilities with our expert guide!
Enhancing Search Functionality in PHP
Creating an efficient and aesthetically pleasing live search feature can significantly enhance the user experience of your website. In this section, we will delve into the full code of search.php and explore ways to optimize it for improved performance.
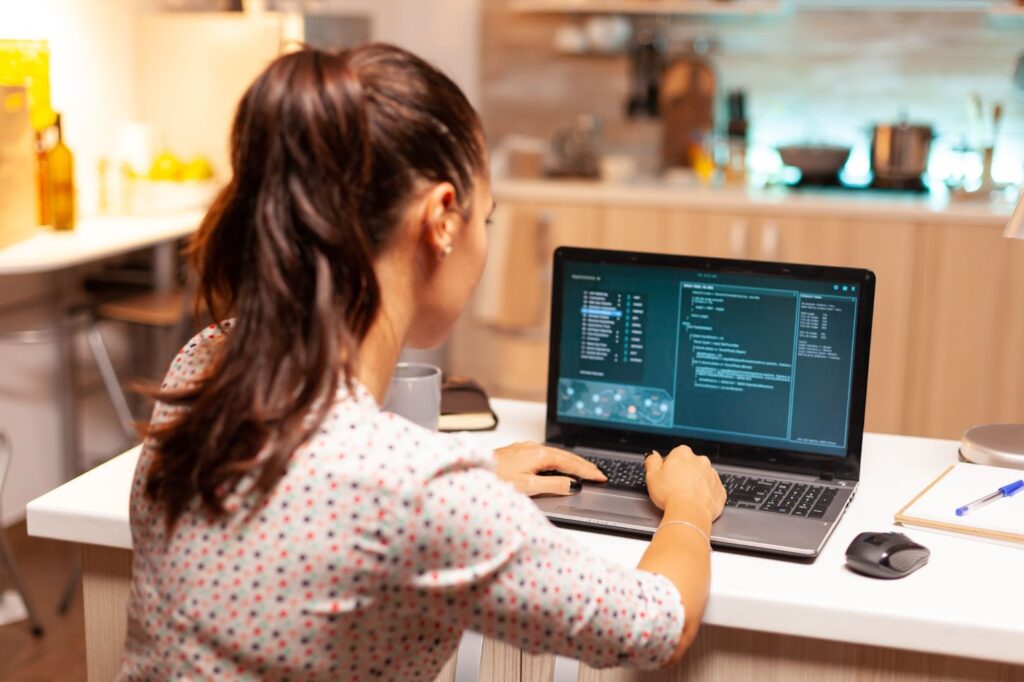
Full Code of search.php
Below is the complete PHP code for search.php, which is responsible for handling user queries and returning search results from a database.
<?php
// Database configuration
$host = 'localhost';
$user = 'root';
$password = '';
$database = 'dev_testing';
// Establish a database connection
$mysqli = new mysqli($host, $user, $password, $database);
// Get user input and sanitize it
$username = $_GET['username'];
$startFrom = $_GET['startFrom'];
$username = trim(htmlspecialchars($username));
$startFrom = filter_var($startFrom, FILTER_VALIDATE_INT);
// Create a wildcard search term
$like = '%' . strtolower($username) . '%';
// Prepare and execute the database query
$statement = $mysqli -> prepare('
SELECT name, picture, description
FROM users
WHERE lower(name) LIKE ?
ORDER BY INSTR(title, ?), title
LIMIT 6 OFFSET ?'
);
if (
$statement &&
$statement -> bind_param('ssi', $like, $username, $startFrom) &&
$statement -> execute() &&
$statement -> store_result() &&
$statement -> bind_result($name, $picture, $description)
) {
// Create an array to store search results
$array = [];
while ($statement -> fetch()) {
$array[] = [
'name' => $name,
'picture' => $picture,
'description' => $description
];
}
// Return the results as JSON
echo json_encode($array);
exit();
}
?>
Tips for Optimizing the PHP Search Functionality:
- Database Indexing: Ensure that your database columns used in search conditions are properly indexed to improve query performance;
- Pagination: Implement pagination to display search results in smaller, manageable chunks, reducing server load and improving user experience;
- Error Handling: Enhance error handling to provide informative messages to users in case of any issues with the search functionality;
- Security: Always sanitize and validate user inputs to prevent SQL injection and cross-site scripting (XSS) attacks.
Now, let’s move on to improving the visual aspect of our live search with CSS.
Elevating Aesthetics with CSS
To create an engaging and visually appealing live search interface, CSS plays a crucial role. Let’s add some style to our search box and search results display.
CSS Code for Beautifying Live Search:
#textbox {
padding: 10px;
border-radius: 5px;
border: 0;
box-shadow: 0 0 4px 0 rgba(0, 0, 0, 0.2);
}
.row img {
width: 40px;
height: 40px;
border-radius: 50%;
vertical-align: middle;
}
.row {
padding: 10px;
}
.row:hover {
background-color: #eee;
}
Conclusion
Numerous websites incorporate live search functionality for various purposes. In this tutorial, I will elucidate the process of crafting your own live search feature by employing AJAX, PHP, and MYSQL. Additionally, we have tastefully integrated some CSS elements into our project to enhance its visual appeal. For those seeking a comprehensive comprehension of each PHP and JavaScript statement individually, I highly recommend acquiring the source code, which is conveniently accessible via the link provided at the outset of this article. This code repository boasts an abundance of explanatory comments, facilitating a deeper grasp of the intricacies involved.
Do not hesitate to contribute your thoughts and queries in the comment section below. Your engagement is greatly appreciated, and thank you immensely for investing your time in this tutorial.