In the world of web development, forms serve as a critical tool, providing an interface for users to submit data. One of the common scenarios when developing PHP forms is the stipulation of ‘required’ fields. These fields are crucial and must be filled by users to ensure the significant data isn’t left out, enhancing the effectiveness of data collection.
Using PHP’s Empty() Function to Validate Inputs
One of the critical tools at our disposal is the empty() function in PHP used to assess whether an input is empty. This function will return true in the following instances:
- When the form is presented with an empty string, represented as “”;
- When the submitted integer is 0;
- In the case of 0.0, which is 0 represented as a float;
- With ‘0’ as a string;
- When the input is null;
- If the input is false;
- With an empty array, represented as [];
Integrating Form and Handler in a Single Script
In practice, you might often find the form and its handler positioned in the same script. This arrangement allows error messages to be displayed directly within the forms, guiding users effectively in their form completion process.
Important Tips and Insights
Remember, data from non-form elements (like <div>, <span>, etc.) enclosed within a form will not be submitted. Hence, while designing your form, ensure that all required data collecting fields are actual form inputs.
PHP Form Validation: The Essential Practice
In PHP, one of the crucial aspects is the use of mandatory input fields. The following code snippet provides an illustrative example of how PHP code could be written to handle required fields.
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
// Prepare to process form data
// Initialize name and email variables
$username = $useremail = '';
// Check if name field is empty
if (empty($_POST['name'])) {
$nameError = 'This field cannot be left empty. Please enter your name.';
} else {
$username = sanitizeInput($_POST['name']);
}
// Check if email field is empty
if (empty($_POST['email'])) {
$emailError = 'We need your email. Please enter a valid email address.';
} else {
$useremail = sanitizeInput($_POST['email']);
}
}
function sanitizeInput($data) {
$data = trim($data);
$data = htmlspecialchars($data);
return $data;
}
?>
<!DOCTYPE html>
<html>
<head>
<title>Form Handling in PHP</title>
<style>
.error-message {
color:red;
}
</style>
</head>
<body>
<form action="" method="post">
Full Name: <input type="text" name="name">
<span class="error-message"><?php if (isset($nameError)) echo $nameError ?></span>
<br>
Email Address: <input type="text" name="email">
<span class="error-message"><?php if (isset($emailError)) echo $emailError ?></span>
<br>
<input type="submit" name="send" value="Submit">
</form>
</body>
</html>
The first part of the script checks if the form has been submitted using the $_SERVER[‘REQUEST_METHOD’] variable. If the form has been submitted (i.e., the request method is POST), it then proceeds to validate the inputs.
Specifically, it checks if the ‘name’ and ‘email’ fields are empty. If either of them is empty, it stores an error message in the relevant variable ($nameError or $emailError). If the fields are not empty, it sanitizes the input data by trimming any whitespace from the beginning or end of the submitted data and using the htmlspecialchars() function to prevent XSS (cross-site scripting) attacks.
The form itself is pretty straightforward, with a text field for the name and email, and an error message positioned near each input field. The error message is displayed only if the corresponding error variable is set, i.e., if the form has been submitted without filling that particular field. The form uses POST as the method and submits the data to the same script.
This is an effective way of validating form inputs and giving immediate feedback to users, enhancing their experience on your site by ensuring they understand exactly what information is expected from them.
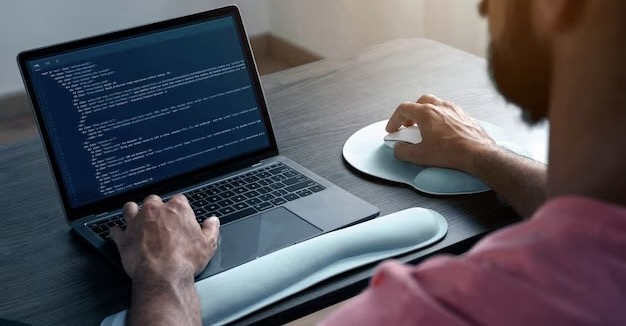
Expanding Horizons: NodeList to Array in JavaScript
As we delve deeper into web development, it’s worth expanding our horizons to explore additional topics that complement our understanding of form handling. One such topic is converting a NodeList to an array in JavaScript. This proficiency can be invaluable when manipulating and processing data gathered from HTML elements.
In many scenarios, web developers work with collections of HTML elements represented as NodeLists. While NodeLists have their utility, they lack the flexibility and functionality of arrays in JavaScript. Converting a NodeList to an array empowers developers with an array’s comprehensive range of methods and features, facilitating more efficient data manipulation and manipulation. This skill becomes particularly useful when dealing with dynamic web content and user interactions, enriching the overall user experience.
Conclusion
Mastering the use of required fields in PHP forms can greatly enhance the data collection process. This holds true not only for the data integrity but also for the user experience. Clear error messages, consistency in error display, and correctly highlighted required fields are crucial practices to follow. As you continue to develop your PHP forms, remember to keep the user experience front of mind for the most effective interactions.