Let’s consider a scenario where you possess a basic blog comprising three fundamental components: the header, the body, and the footer. Now, envision a situation where you need to modify the header on every page. Would you embark on the tedious task of opening and altering each individual page file manually? Certainly not. Instead, you can efficiently manage this by maintaining the header in a single file and incorporating it into the remaining files.
In essence, the techniques of including and requiring serve as invaluable tools for reusing PHP files. They bestow upon us the remarkable ability to streamline project management, permitting us to effect changes to the entire layout by simply editing a solitary file. Within the scope of this chapter, we shall delve into the art of integrating the content from one PHP file seamlessly into another PHP file.
Mastering PHP File Inclusion: A Comprehensive Guide
When it comes to including files in PHP, you have four powerful tools at your disposal: include, require, include_once, and require_once. Each of these statements serves a unique purpose and offers distinct advantages. In this comprehensive guide, we’ll delve deep into each statement, exploring their differences, use cases, and best practices.
The Four Mighty Statements
PHP provides four essential statements for including files:
- include;
- require;
- include_once;
- require_once.
include vs. require: Unveiling the Differences
At first glance, include and require may seem identical, but they differ significantly in their error-handling behavior when a file is not found. Understanding these differences is crucial for crafting robust PHP applications.
- include
- Behavior: include produces a warning if the specified file is not found but continues executing the script;
- Use Cases: Recommended when the included script is not critical to the script’s execution flow.
- require
- Behavior: require goes a step further by producing a fatal error if the file is not found, effectively halting script execution;
- Use Cases: Should be used when the included script is essential for the subsequent processes to function correctly.
Adding _once for Precision
For those who demand precision in file inclusion, PHP offers the option to add _once to both include and require. This addition introduces a new rule: “Include the file only if it is not already included.”
- include_once
- Behavior: When you use include_once, PHP checks if the file has already been included in the current script. If not, it includes the file; otherwise, it skips the inclusion;
- Best Practice: Consider using include_once as it prevents multiple inclusions of the same file, ensuring script efficiency and preventing errors.
- require_once
- Behavior: Similar to include_once, require_once checks if the file has already been required or included. If not, it requires the file; otherwise, it skips the inclusion;
- Best Practice: Ideal when you want to ensure a file is only included once and stop script execution if the file is not found. Particularly useful for maintaining code integrity.
How to Use PHP Include and Require Statements Effectively
In the world of web development, managing code efficiently is crucial. PHP developers often find themselves needing to reuse parts of code across multiple pages to maintain consistency and improve productivity. Two essential PHP functions, include and require, come to the rescue. These functions allow you to insert the content of one PHP file into another, streamlining your code and enhancing maintainability.
Let’s delve into how to use these functions effectively, exploring examples and best practices:
1. The include Function
The include function is used to embed the content of one PHP file within another. It’s particularly useful for incorporating reusable elements like headers, footers, or navigation menus across multiple web pages. Here’s how to use it:
Example: PHP include
<html>
<body>
<?php include 'header.php' ?>
<p>I'm using the include statement</p>
<?php include 'footer.php' ?>
</body>
</html>
Place the include statement where you want to include the external file.
If the included file doesn’t exist, PHP will produce a warning and continue executing the script.
2. The require Function
The require function works similarly to include but with a critical difference: it treats the inclusion as mandatory. If the specified file isn’t found, PHP will generate a fatal error and halt script execution. Use require when you absolutely need a file to be included for the page to function correctly:
Example: PHP require
<html>
<body>
<?php require 'header.php' ?>
<p>I'm using the require statement</p>
<?php require 'footer.php' ?>
</body>
</html>
Employ require when the included file is crucial for the page to work correctly. It’s a best practice to use require for files that contain essential functions or configurations.
3. The include_once Function
When you use the include function multiple times in the same script, there’s a possibility of unintended behavior or errors. The include_once function solves this problem by ensuring that the specified file is included only once during script execution:
Example: PHP include_once
<html>
<body>
<?php include_once 'header.php' ?>
<p>I'm using the include_once statement</p>
<?php include_once 'footer.php' ?>
<?php include_once 'footer.php'; // this has no effect, preventing duplication ?>
</body>
</html>
Use include_once to avoid redundancy and potential issues when including the same file multiple times.
4. The require_once Function
Similar to include_once, the require_once function ensures that a file is included only once. It’s especially useful when you want to guarantee the inclusion of a file without duplicates:
Example: PHP require_once
<html>
<body>
<?php require_once 'header.php' ?>
<p>
I'm using the require_once statement for the header and include for the footer.
No matter what we used, require_once checks if the file was included or required earlier.
So, the last require_once has no effect.
</p>
<?php include 'footer.php' ?>
<?php require_once 'footer.php'; // this has no effect, avoiding redundancy ?>
</body>
</html>
require_once is ideal when you need to ensure the inclusion of critical files without risking duplicates.
It helps maintain code integrity by preventing unintended file inclusions.
Best Practices for Including and Requiring Files in PHP
In PHP, the use of include and require statements is pivotal for incorporating external files into your projects. Whether you’re working with PHP for web development, application programming, or any other use case, mastering these techniques is essential for a seamless workflow. Here, we’ll delve into some valuable tips and insights for including and requiring files in PHP.
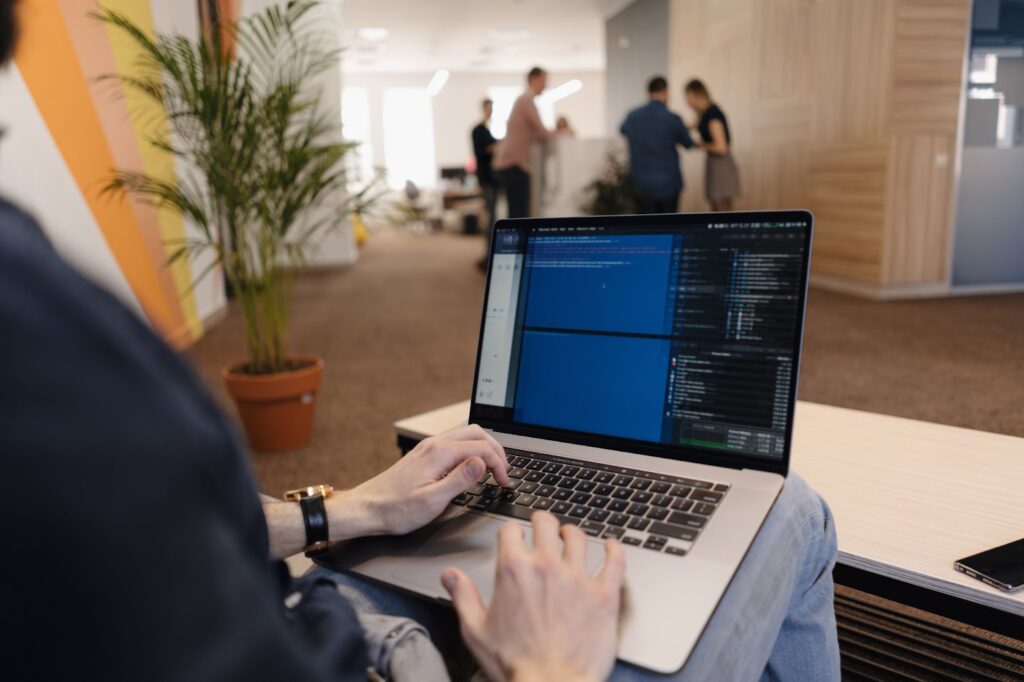
1. Understanding the Basics
Before diving into the intricacies, let’s clarify the fundamental concepts:
- include and require: Both statements are used to include files in PHP. The primary difference is that if require fails to include the specified file, it will result in a fatal error, whereas include will produce a warning and continue execution;
- _once suffix: Adding _once to either include or require ensures that the file is included only once, even if the statement is encountered multiple times in your code.
2. Absolute vs. Relative Paths
When including or requiring files, you have the flexibility to specify paths using either absolute or relative references. Here’s how to navigate both options effectively:
Absolute Paths: Utilize $_SERVER[‘DOCUMENT_ROOT’] to start from the document root. This approach ensures that you’re accessing files from a consistent and predictable location. For example:
require_once $_SERVER['DOCUMENT_ROOT'] . '/folder/file.php';
Relative Paths: Employ __DIR__ to reference the current file’s directory. Combine it with /../ to traverse previous folders as needed. This method offers flexibility in locating files relative to the current script:
require_once __DIR__ . '/file-in-this-folder.php';
require_once __DIR__ . '/../file-in-previous-folder.php';
require_once __DIR__ . '/../../file-in-two-folders-back.php';
3. Security Considerations
One critical aspect of file inclusion is security. Always prioritize the safety of your application or website by following these guidelines:
Avoid Including User-Uploaded Files: Never include or require files that have been uploaded by users, especially if they contain executable PHP code. This practice can lead to severe security risks. For instance, if a user uploads a .css file containing malicious PHP code, all of it will execute when included or required.
Conclusion
In conclusion, by adopting the practice of including and requiring PHP files, we have unlocked a powerful mechanism for enhancing efficiency and simplicity in managing web projects. The ability to centralize elements such as headers and reuse them across multiple pages not only saves time but also promotes consistency and ease of maintenance. As we have explored in this chapter, the art of seamlessly integrating PHP file content into others opens up a world of possibilities for creating dynamic and cohesive web applications. This skill is an essential tool in the toolkit of any web developer seeking to create robust and maintainable websites.