Website security is paramount in today’s digital world. One prevailing threat is Cross-Site Scripting (XSS) attacks, which can compromise site security by injecting malicious scripts into web pages viewed by other users. These attacks could potentially steal sensitive data, manipulate web content, or perform unwanted actions on behalf of the victimized users.
To mitigate such risks, it’s vital to escape HTML. PHP is equipped with an in-built function, htmlspecialchars(), that makes this task a cakewalk. This function converts predefined characters with special meaning in HTML to their corresponding HTML entities, preserving their significance while eliminating the risk of XSS attacks.
Let’s illustrate this with an example. If a web application doesn’t check and filter input properly, a hacker can embed a script like <script>alert(‘Hacked’)</script>. In its raw form, this script is an XSS attack waiting to happen.
However, when passed through the htmlspecialchars() function, the script is rendered harmless, transforming into &lt;script&gt;alert('Hacked')&lt;/script&gt;.
Here are the conversions htmlspecialchars() function makes:
- & becomes &amp;
- < becomes &lt;
- > becomes &gt;
- ” becomes &quot;
- ‘ becomes &#039;
This handy function ensures that these symbols only get interpreted as display characters, not as HTML tags or entities.
Example of htmlspecialchars() in Action
Consider the following PHP code snippet:
<?php
$string = '<script>alert("Hello")</script>';
echo htmlspecialchars($string);
Reinforcing Web Security: Verifying Request Methods in PHP
Building robust and secure web applications requires diligent attention to various factors, one of which is validating the request method. This process ensures that the server only responds to the correct type of request made by a client. In PHP, you can reference the global $_SERVER array to access the request method.
When developing a web application, you may choose to design a form that uses the POST method to send data. It is important in such situations to confirm the form’s submission using the specific method intended for it.
The following PHP code snippet provides an example of how to validate a request method:
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
// valid request
} else {
die('Invalid Request');
}
This code block uses the $_SERVER[‘REQUEST_METHOD’] to access the request method information. The if statement then checks if the request method is POST. If the condition is met, the script continues execution as expected for a valid request.
However, if the request method is not POST, the die() function is called with ‘Invalid Request’ as an argument. The die() function is a standard PHP feature that halts script execution and outputs any parameters passed to it. In this case, an ‘Invalid Request’ message will notify developers monitoring the server about the unsuccessful request.
Useful Tips:
- Always validate request methods to ensure your server is secure and data integrity is maintained;
- The die() function is a powerful tool in any PHP developers’ toolkit. It helps in debugging and ensures the script doesn’t proceed when abnormal conditions are detected;
- Remember, the $_SERVER[‘REQUEST_METHOD’] provides the request method type, which can be GET, POST, PUT, DELETE, etc. Be sure to validate it according to your specific use-case scenario.
Optimizing Text Output: Eradicating Extra White Spaces with PHP
When dealing with string data in PHP, it is quite common to encounter unwanted white spaces at the beginning or end of the string. These superfluous spaces can cause issues when comparing strings, storing data, or rendering output for end-users. Thankfully, PHP offers a handy function, trim(), which can efficiently remove these unnecessary white spaces.
An Overview of the trim() Function
The trim() function in PHP is specifically designed to remove white spaces from the beginning and end of a string. White spaces include spaces, newlines, horizontal tabs, and other similar characters. The function leaves the interior spacing of a string intact, focusing only on leading and trailing white spaces.
Below is the syntax for the trim() function:
$string = trim($input);
In this syntax, $input refers to the string you wish to trim. The function returns a new string, without leading or trailing white spaces, which can be assigned to the $string variable.
Walking Through an Example
Consider the following PHP code:
<?php
$text = ' Hello ';
echo $text; // Outputs: ' Hello '
echo '<br>';
echo trim($text); // Outputs: 'Hello'
?>
In this example, $text initially contains white spaces at the beginning and end. After passing $text through the trim() function, the leading and trailing white spaces are removed.
Key Insights and Recommendations:
- Utilize the trim() function to eliminate unwanted white spaces, enhancing data integrity and consistency;
- Remember that trim() only removes white spaces at the beginning and end of a string while preserving the internal spacing;
- For a more specific trimming operation, PHP also offers ltrim() to remove leading white spaces and rtrim() to remove trailing white spaces.
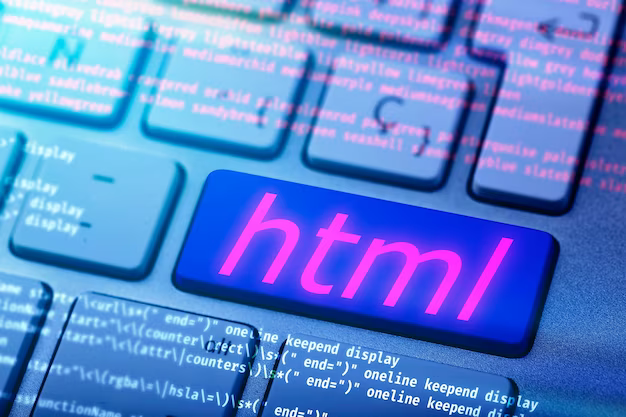
Euclidean Distance in JavaScript
While PHP excels in addressing server-side security concerns, JavaScript plays a pivotal role in enhancing the user experience on the client side. One fundamental concept in JavaScript is Euclidean Distance, often utilized in data analysis and visualization. It measures the geometric distance between two points in a multidimensional space.
The integration of Euclidean Distance in JavaScript can be particularly valuable in scenarios where you need to calculate distances between data points, such as clustering similar items, recommending products, or implementing location-based services. This mathematical tool can be leveraged to find similarities or dissimilarities between vectors, aiding in decision-making processes.
Conclusion
The htmlspecialchars() function is a powerful tool for protecting your PHP applications from XSS attacks. By understanding how this function works, including its parameters and flag values, you can effectively sanitize user input and maintain your application’s integrity. As with any tool, it’s crucial to follow best practices, like specifying the correct encoding and understanding how to handle quotes correctly. Mastering the use of htmlspecialchars() is a significant step towards improving your PHP coding skills and securing your web projects.