Variables in PHP have the capability to store a variety of data types, with PHP automatically determining the appropriate data type for a variable. This flexibility is especially useful as we often need to store diverse data types in variables to accomplish various tasks. PHP offers a range of data types, including Boolean, Integer, Float (or Double), String, Array, Object, and Null, allowing developers to work with data in a versatile and efficient manner.
In the realm of PHP Data Types, understanding default arguments in PHP is a crucial aspect that enhances the flexibility and functionality of functions and methods within your code.
PHP’s var_dump() Function for Variable Inspection
The var_dump() function in PHP is a versatile tool for inspecting variables. Its primary purpose is to provide detailed information about a variable, including its data type and current value. This function plays a crucial role in debugging and understanding the contents of variables in your PHP code. Similar to the echo() function, var_dump() is an output function, but it goes a step further by offering comprehensive insights into your data.
Let’s illustrate how var_dump() works with a simple example:
<?php
$x = 12;
var_dump($x);
?>
In this example, the var_dump() function is applied to the variable $x, revealing its data type (integer) and value (12). This information can be invaluable when working with complex data structures or when troubleshooting issues in your PHP scripts.
Boolean Data Type in PHP
In PHP, a Boolean represents a fundamental data type that can assume one of two distinct values: true or false. Booleans are a cornerstone of programming logic, enabling you to make decisions and control the flow of your code based on conditions.
The Boolean data type in PHP is exceptionally straightforward, as it only accommodates the values true or false. These values are not case-sensitive, meaning you can use either “true” or “false” in lowercase or uppercase.
Let’s explore a practical example of working with Booleans in PHP:
<?php
$a = true; // assign true to $a
$b = false; // assign false to $b
var_dump($a);
var_dump($b);
Integer Data Type in PHP
In PHP, an integer represents a whole number, which means it is not a fraction or a decimal but a whole numerical value (e.g., -2, -1, 0, 1, 2, 3, …). Integers can be either positive or negative, and they play a fundamental role in performing arithmetic operations and storing numerical data.
There are several ways to specify integers in PHP, each with its own notation:
- Decimal Notation (Base 10): Integers in their standard form, such as 1, 42, or -100, are represented in decimal notation, which is the base 10 system;
- Hexadecimal Notation (Base 16): Hexadecimal notation uses the 0x prefix followed by a series of hexadecimal digits (0-9 and A-F), like 0x1A or 0xFF;
- Octal Notation (Base 8): Octal notation uses the 0 prefix followed by octal digits (0-7), like 075 or 011;
- Binary Notation (Base 2): Binary notation uses the 0b prefix followed by a sequence of binary digits (0 and 1), like 0b1101 or 0b1010.
Here’s a table illustrating how to specify the integer 128 in various notations:
Notation | Representation |
---|---|
Decimal (Base 10) | 128 |
Hexadecimal (Base 16) | 0x80 |
Octal (Base 8) | 200 |
Binary (Base 2) | 0b10000000 |
Let’s explore how to work with integers in PHP by examining a practical example:
<?php
// 128 in different notations
$a = 128; // decimal number
var_dump($a);
$a = -128; // a negative number
var_dump($a);
$a = 0x80; // hexadecimal number
var_dump($a);
$a = 0200; // octal number
var_dump($a);
$a = 0b10000000; // binary number
var_dump($a);
As a beginner, you do not need to memorize hexadecimal, octal, and binary notations right away.
Floating-Point Numbers in PHP
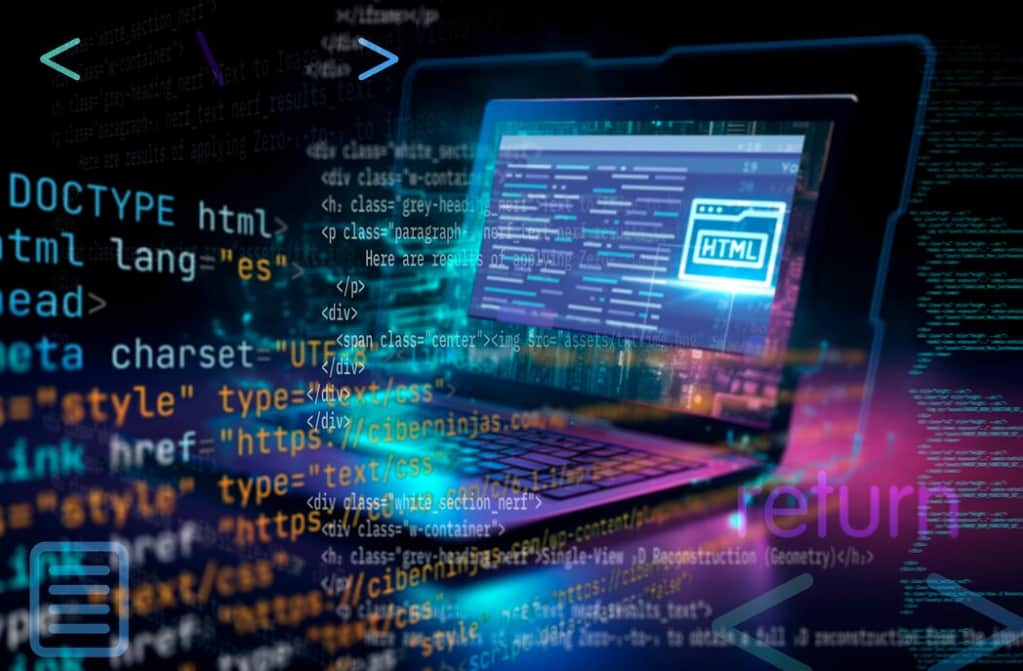
In PHP, a float is a numeric data type that can represent numbers with fractional parts. Floats are versatile and are often used to handle values like 2.56, 1.24, or scientific notations like 7E-10. They are also referred to as “Double,” “Real,” or “Floating-Point Numbers.”
Let’s take a look at a practical example of using floats in PHP:
<?php
$a = 2.56;
var_dump($a);
$a = 2.56e3; // 2.56 multiplied by 3rd power of 10
var_dump($a);
$a = 2.56e-5; // 2.56 multiplied by -5th power of 10
var_dump($a);
String Data Type in PHP
In PHP, a string is a fundamental data type that represents a finite sequence of characters, including letters, numerals, symbols, punctuation marks, and more. Strings are indispensable in programming, as they allow you to work with textual data, manipulate text, and create dynamic content.
There are four primary ways to specify a string in PHP:
- Single Quoted: Strings enclosed in single quotes, like ‘Hello, World!’. In single-quoted strings, escape sequences are not interpreted, and variables are not interpolated;
- Double Quoted: Strings enclosed in double quotes, like “Hello, World!”. Double-quoted strings allow for the interpretation of escape sequences and variable interpolation;
- Heredoc Syntax: A special syntax for specifying strings, useful for multiline strings. It starts with <<< followed by an identifier, followed by the string content, and terminated by the same identifier;
- Nowdoc Syntax: Similar to heredoc, but behaves like single-quoted strings, meaning variables are not interpolated. It starts with <<<‘identifier’, followed by the string content, and terminated by the same identifier.
Let’s explore a simple example of using different string representations in PHP:
<?php
$str = 'This is single quoted';
$str = "This is double quoted";
$str = <<<EOD
This is a heredoc example
This can be multiline
EOD;
$str = <<<'EOD'
This is a nowdoc example
This can be multiline
EOD;
var_dump($str);
Array Data Type in PHP
In PHP, an array is a fundamental data structure used to store and manage a series of values. Arrays provide a versatile way to organize and manipulate data, and they can hold elements of various types, including numbers, strings, and even other arrays.
There are two common ways to declare an array in PHP:
- Using the array() function: You can declare an array by using the array() function and specifying its elements within the parentheses, separated by commas;
- Using square brackets [ and ]: Alternatively, you can declare an array by wrapping its elements with square brackets [ and ]. This is a more concise and modern way to create arrays in PHP.
Elements within an array should be separated by commas, and you can include elements of any data type covered in this chapter, making arrays highly flexible and powerful data structures.
Let’s explore a basic example of declaring and inspecting arrays in PHP:
<?php
$array = array('Apple', 'Banana', 'Orange', 'Mango');
var_dump($array);
$array = ['Apple', 'Banana', 'Orange', 'Mango'];
var_dump($array);
PHP Objects
In PHP, an object represents a specific instance of a class. An object can be thought of as a composite structure that combines variables, functions, and data structures into a single entity. Objects are fundamental to object-oriented programming (OOP) in PHP, allowing you to create reusable and organized code by modeling real-world entities as classes and then creating instances of those classes as objects.
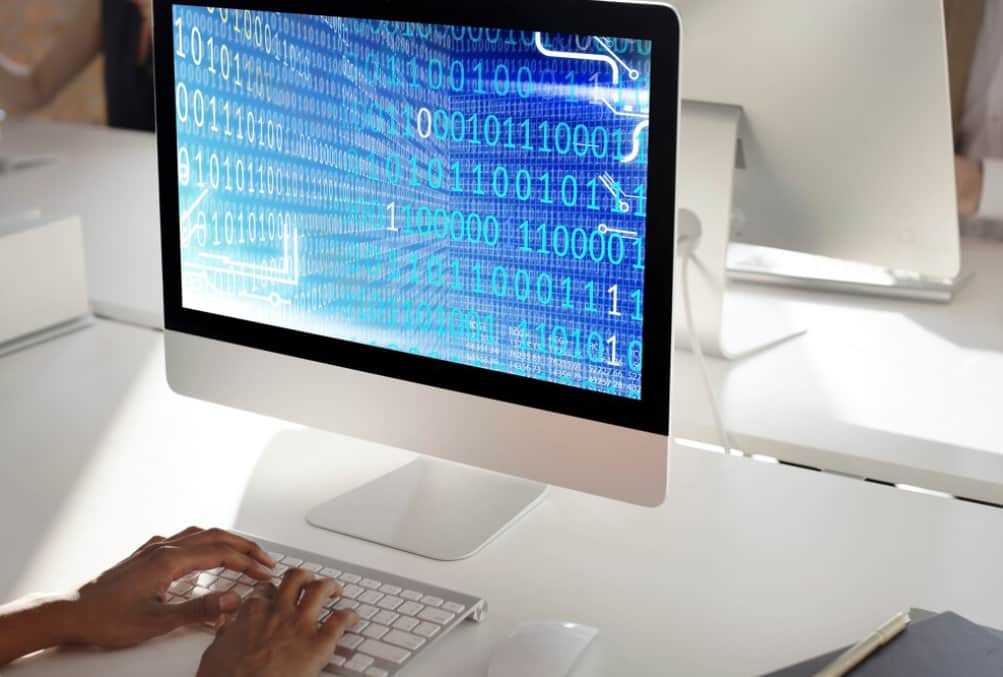
Null in PHP
In PHP, null is a special value that signifies the absence or lack of a value in a variable. It is used to represent situations where a variable has no assigned value or is intentionally left empty. Null is distinct from other values such as 0 or false; it is a unique state that indicates the absence of any data.
It’s important to note that null in PHP is case-insensitive. Whether you use “Null,” “null,” or “NULL,” they are all considered equivalent and represent the null value.
Let’s explore an example to illustrate the concept of null in PHP:
<?php
$var = null;
var_dump($var);
// unsetting variables with null
$text = 'Hello World';
$text = null; // now $text does not hold any value
var_dump($text);
Conclusion
PHP data types are the backbone of PHP programming, enabling the creation of dynamic websites and applications. By understanding these fundamental data types – Boolean, Integer, Float, String, Array, Object, and Null – and their practical application, one can truly leverage the power of PHP. Whether it’s using the var_dump() function for data inspection, handling Boolean logic, manipulating numerical data with Integers and Floats, or working with complex data structures like Arrays and Objects, mastery of these data types can facilitate effective problem-solving and efficient code writing.