Introduction to PHP Superglobal Variables
Superglobal variables in PHP are foundational structures always accessible no matter what the scope. On a global scale, they can be used directly inside functions, as exhibited by the $GLOBALS array explored in previous discussions. This accessibility stems from their ‘superglobal’ status, translating to broad availability throughout the script.
If you’re interested in the Super Global Array in PHP, you may also like learning about namespaces in PHP, which provide a way to group related classes and functions, further streamlining and organizing your code.
PHP’s Nine Superglobals
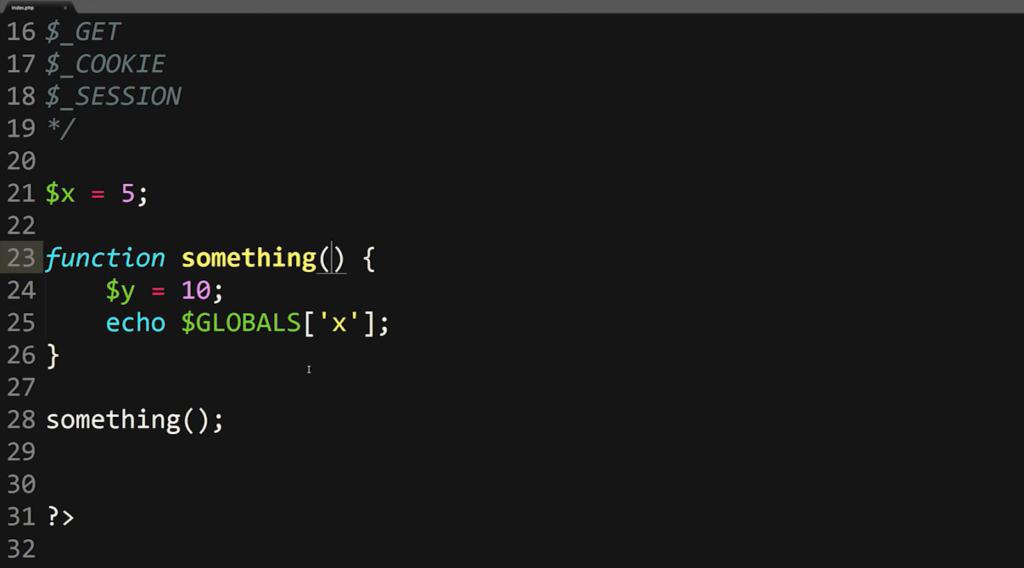
There are nine PHP superglobal variables, each essentially acting as an indexed or associative array holding specific data sets. These include:
- $GLOBALS;
- $_SERVER;
- $_GET;
- $_POST;
- $_FILES;
- $_ENV;
- $_COOKIE;
- $_SESSION;
- $_REQUEST.
Notably, $GLOBALS is the only superglobal variable that doesn’t follow the _$ (underscore after the dollar sign) naming convention.
Each of these superglobals has a specific role in the PHP ecosystem, which may be somewhat overwhelming to remember initially. With practice, however, their functions become more intuitive.
Superglobal Variable | Description |
---|---|
$GLOBALS | Stores all the global scope variables. |
$_SERVER | Stores information about the server and execution environment. |
$_GET | Stores HTTP GET variables. |
$_POST | Stores HTTP POST variables. |
$_FILES | Stores HTTP file upload variables. |
$_ENV | Stores environment variables. |
$_COOKIE | Stores HTTP Cookies. |
$_SESSION | Stores session variables. |
$_REQUEST | Stores request variables ($_GET, $_POST and $_COOKIE variables). |
An Overview of $GLOBALS
The $GLOBALS superglobal array serves as a repository for all globally scoped variables. Here’s an example to illustrate:
<?php
$x = 'Hyvor';
$y = 'Developer';
function websiteName() {
echo $GLOBALS['x'], $GLOBALS['y'];
}
websiteName(); // outputs HyvorDeveloper
An Understanding of $_SERVER
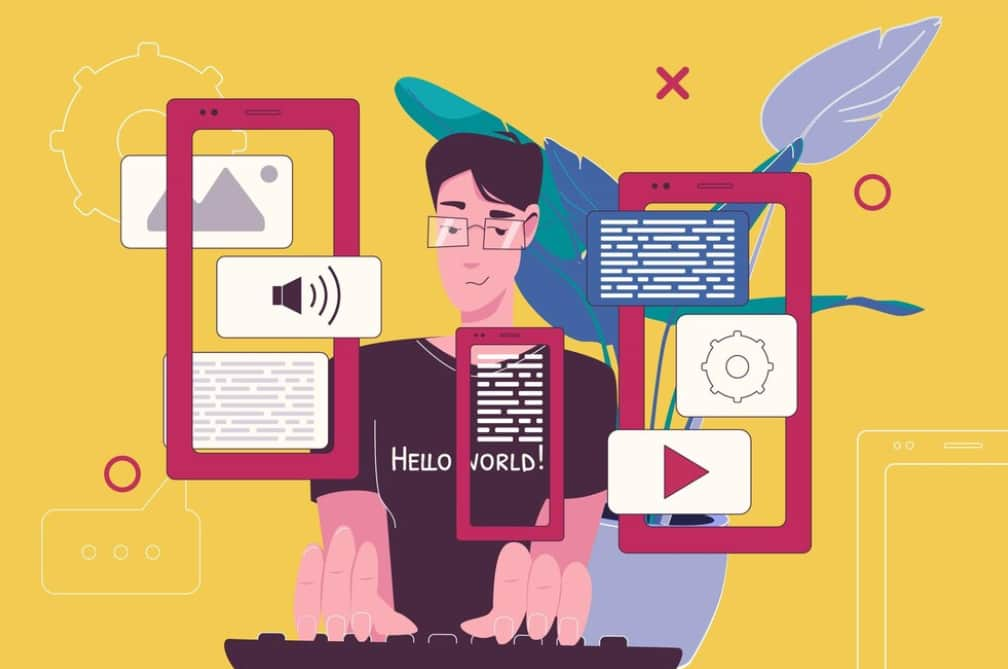
The $_SERVER superglobal array is a comprehensive container of information regarding the currently running script, network addresses, paths, locations and more. The PHP manual offers a detailed inventory of available elements within the $_SERVER superglobal array. This guide will only touch on the commonly used ones.
Element | Description |
---|---|
PHP_SELF | The filename of the currently executing script, relative to the document root. |
DOCUMENT_ROOT | The document root directory of the server. |
SERVER_ADDR | The IP address of the server. |
SERVER_NAME | The name of the server. (For instance, developer.hyvor.com) |
REQUEST_METHOD | The request method that the page was requested with. (Ex: POST, GET, HEAD, etc.) |
REQUEST_TIME | The timestamp of the start of the request. |
HTTP_USER_AGENT | The User-Agent header sent by the browser. (This is used to detect OS, Browser, etc.) |
REMOTE_ADDR | The IP address of the current user. |
Example of usage:
<?php
echo $_SERVER['PHP_SELF'] . '<br>';
echo $_SERVER['DOCUMENT_ROOT'] . '<br>';
echo $_SERVER['SERVER_ADDR'] . '<br>';
echo $_SERVER['SERVER_NAME'] . '<br>';
echo $_SERVER['REQUEST_METHOD'] . '<br>';
echo $_SERVER['REQUEST_TIME'] . '<br>';
echo $_SERVER['HTTP_USER_AGENT'] . '<br>';
echo $_SERVER['REMOTE_ADDR'];
Conclusion
PHP superglobal arrays are undeniably powerful tools in web development. Their versatility and wide-scale accessibility allow developers to manage a plethora of functionalities, from accessing global variables to storing HTTP and server information. Mastering their use can significantly boost your PHP development skills, enabling you to create more dynamic and user-centric applications. Remember, it’s not about memorizing each superglobal, but understanding their purpose and how they can be manipulated to enhance your web application operations.