In the realm of MySQLi, prepared statements take center stage in a multitude of operations. Despite their prevalence, truly comprehensive guides that shed light on their most effective application are few and far between. This article aims to bridge that knowledge gap by diving deep into the world of MySQLi prepared statements, unveiling the simplest and most effective methods to utilize them while negating the potential risks of SQL Injection.
An Introduction to Prepared Statements in MySQLi
As developers leverage SQL queries to communicate with databases, bad actors have exploited this interaction through SQL Injections, leading to serious security concerns. Prepared statements were formulated as a solution to this prevalent problem. Here’s the mechanism behind how they function:
- An SQL query void of variables is transmitted to the database. The symbol “?” is designated as the placeholder for these variables;
- Following the initial query, variables are sent separately to the database, tagged with a distinct message. This message effectively communicates to the database to interpret these variables as simple data inputs and not SQL commands;
- Subsequently, the initial query can be executed with a myriad of varying variables.
To illustrate the simplicity and effectiveness of this defensive measure, let’s explore the execution of a basic SELECT MySQLi prepared statement in PHP. For this example, make sure to substitute HOST, USERNAME, PASSWORD, and DATABASE with your personal database credentials.
//Establishing connection with the database
$mysqli = new mysqli(HOST, USERNAME, PASSWORD, DATABASE);
//Designating a variable
$id = 2;
//Preparing the statement
$stmt = $mysqli -> prepare('SELECT email FROM users WHERE id = ? LIMIT 1');
$stmt -> bind_param('i', $id);
$stmt -> execute();
$stmt -> store_result();
$stmt -> bind_result($email);
$stmt -> fetch();
//Outputting the result
echo $email;
If executed successfully, the script will display the email associated with the user who possesses the id “2”. However, this approach harbors a significant flaw…
Effectively Implementing Prepared Statements
In the vast landscape of SQL database management, programmers must always be on the lookout for potential failure points within their scripts. To ensure effective execution and maximum protection against SQL injection, programmers should validate that every step in their MYSQLI prepared statement is successfully completed before proceeding to the next.
Consider the following script which does just that:
// Designating a variable
$id = 2;
// Preparing the statement
$stmt = $mysqli -> prepare('SELECT email FROM users WHERE id = ? LIMIT 1');
// If the preparation and execution of the statement are successful, print the email
// Otherwise, print an error message
if (
$stmt &&
$stmt -> bind_param('i', $id) &&
$stmt -> execute() &&
$stmt -> store_result() &&
$stmt -> bind_result($email) &&
$stmt -> fetch()
) {
echo $email;
} else {
echo 'Prepared Statement Error';
}
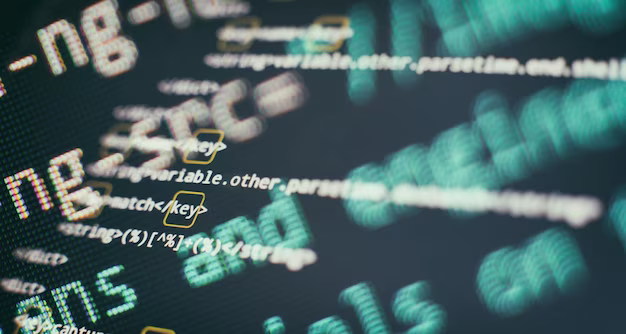
Comprehensive Examples for Enhanced MySQLi Understanding
Multi-row Selections
The power of MySQLi prepared statements extends beyond retrieving individual rows — they can also return multiple rows as a multidimensional array.
Suppose we have a database table with numerous records of user data (including name and email). Here’s how you can select and display the names and emails of users with the role of “admin”:
$role = 'admin';
$stmt = $mysqli -> prepare('SELECT name, email FROM users WHERE role = ?');
if (
$stmt &&
$stmt -> bind_param('s', $role) &&
$stmt -> execute() &&
$stmt -> store_result() &&
$stmt -> bind_result($name, $email)
) {
while ($stmt -> fetch()) {
echo "$name: $email <br>";
}
} else {
echo 'Prepared Statement Error';
}
The while loop in this snippet continues to execute as long as the fetch() method returns ‘true’, i.e., until there are no more rows to retrieve. On each iteration, the $name and $emailvariables are updated with the values of the current row.
Using INSERT Statement
Here’s how you can conveniently insert multiple rows using the same prepared statement:
$mysqli = new mysqli(HOST, USERNAME, PASSWORD, DATABASE);
$stmt = $mysqli -> prepare('INSERT INTO users (name, email) VALUES (?,?)');
function insertUser($name, $email) {
global $stmt;
if (
$stmt &&
$stmt -> bind_param('ss', $name, $email) &&
$stmt -> execute()
) {
// New user added
}
}
insertUser('George', '[email protected]');
insertUser('Jack', '[email protected]');
Here, a global prepared statement is opened, followed by the definition of a function insertUser that inserts different variable values to the same prepared statement. This is an excellent way to insert multiple rows into the MySQL database at once.
Update Operation
Update operations are quite similar to inserts, as illustrated in the example below:
$mysqli = new mysqli(HOST, USERNAME, PASSWORD, DATABASE);
$stmt = $mysqli -> prepare('UPDATE users SET name = ? WHERE id = ? LIMIT 1');
$name = 'Joe';
$id = 1;
if (
$stmt &&
$stmt -> bind_param('si', $name, $id) &&
$stmt -> execute() &&
$stmt -> affected_rows === 1
) {
echo 'Updated';
} else {
echo 'Not updated';
}
Debugging: An Essential Tool for Programmers
Programmers are no stranger to debugging—it’s an integral part of code development and management. Debugging SQL, particularly MYSQLI statements, is crucial as this is a common area where errors can occur.
Each MYSQLI variable (e.g., $mysqli in our examples) and statement variable (like $stmt in our examples) contain a vital error property (i.e., $mysqli -> error and $stmt -> error). Knowledge of these properties is one’s first step towards proficient debugging.
Understanding the Use of $mysqli -> error
Let’s say that you have a piece of code that should be updating your database, but it isn’t.
$mysqli = new mysqli(HOST, USERNAME, PASSWORD, DATABASE);
$stmt = $mysqli -> prepare('UPDATE users SET name = ? WHERE id = ? LIMIT 1');
$name = 'Joe';
$id = 1;
if ($stmt && $stmt -> bind_param('si', $name, $id) && $stmt -> execute() && $stmt -> affected_rows === 1) {
echo 'Updated';
} else {
echo 'Not updated';
}
Instead of wasting precious hours hunting for the error, you can quickly identify it by simply adding echo $mysqli -> error; to the else block.
$mysqli = new mysqli(HOST, USERNAME, PASSWORD, DATABASE);
$stmt = $mysqli -> prepare('UPDATE users SET name = ? WHERE id = ? LIMIT 1');
$name = 'Joe';
$id = 1;
if ($stmt && $stmt -> bind_param('si', $name, $id) && $stmt -> execute() && $stmt -> affected_rows === 1) {
echo 'Updated';
} else {
echo $mysqli -> error;
}
After doing the above, for instance, the system might indicate an error like: “Table ‘usres’ doesn’t exist”. This instantly points out that there has been a typo in naming the table as ‘usres’ instead of ‘users’.
It’s important to note that if there’s an error in the SQL query causing the statement preparation to fail, $stmt -> error cannot be used because $stmt is just a boolean – false, and not an object.
What to Do if $mysqli -> error is not Informative?
In cases where $mysqli -> error does not provide any practical information, it generally means that the prepared statement was prepared correctly. The error could indeed be in the bind_param function or other related functions. In such cases, the error messages are stored in $stmt -> error.
$stmt -> error.
$mysqli = new mysqli(HOST, USERNAME, PASSWORD, DATABASE);
$stmt = $mysqli -> prepare('UPDATE users SET name = ? WHERE id = ? LIMIT 1');
$name = 'Joe';
$id = 1;
if ($stmt && $stmt -> bind_param('si', $name, $id) && $stmt -> execute() && $stmt -> affected_rows === 1) {
echo 'Updated';
} else {
echo $stmt -> error;
}
Now, any error associated with the prepared statement would be echoed out, making it easier to troubleshoot.
Debugging for Live Websites
When it comes to live websites, developers should avoid performing the aforementioned debugging steps. Exposing database error messages could potentially lead hackers to the database. In such cases:
- Double-check the query to ensure it’s free of typos;
- Check that the bind_param function’s first parameter is correct and matches the query’s question mark count;
- Attempt running the query in phpMyAdmin or similar database management software;
- Apply your unique debugging techniques;
- Seek help online if the issue persists.
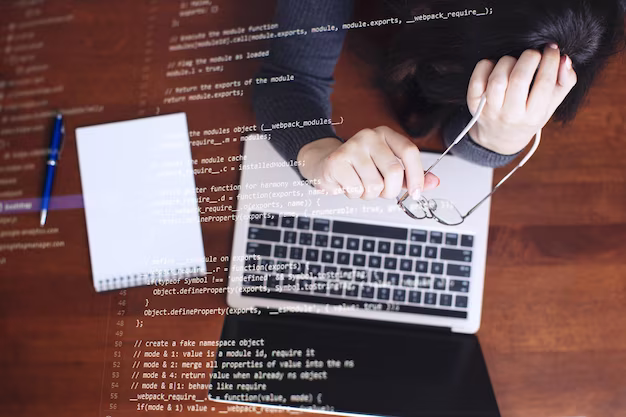
PHP Forms Tutorials: A Synergy with MySQLi Prepared Statements
In the realm of web development, PHP forms tutorials play a pivotal role in user interaction and data manipulation. When harmoniously combined with the power of MySQLi prepared statements, these tutorials form a robust alliance.
Developers can harness the capabilities of PHP forms to gather user input, which is then seamlessly integrated into MySQLi prepared statements. This synergy enables the creation of dynamic, data-driven web applications that not only engage users but also ensure the security and integrity of the data they handle.
By following PHP forms tutorials and incorporating MySQLi prepared statements into your projects, you empower yourself to build web applications that are not only visually appealing but also robust, secure, and resilient to SQL injection attacks. This union of technologies opens up a world of possibilities, allowing you to create interactive websites that respond dynamically to user input while safeguarding your data from potential threats.
Conclusion
To wrap it up, MYSQLI prepared statements and their proper debugging form the backbone of any efficient database operation. The road to mastering these techniques is filled with understanding MYSQLI’s intricacies, digging deep into its functionalities, and keeping an open mind for relentless learning and practice. May your coding journey be filled with explorations, discoveries, and immense gratification.