A PHP operator is a symbol that denotes a specific action or operation.
For instance:
- The ‘+’ symbol is an arithmetic operator for addition;
- The ‘-‘ symbol represents subtraction, also an arithmetic operator.
PHP categorizes its operators into 11 distinct types:
- Arithmetic Operators;
- Assignment Operators;
- Comparison Operators;
- Logical Operators;
- Increment/Decrement Operators;
- String Operators;
- Array Operators;
- Type Operators;
- Bitwise Operators;
- Error Control Operators;
- Execution Operators;
- Spaceship Operator.
This tutorial will focus on the first seven operator types, as they are the most frequently used in PHP programming.
While exploring the concept of variable scope in PHP, it’s important to understand how array operators in PHP can affect the scope of variables in your code.
PHP Basic Arithmetic Operators
These operators are similar to the elementary arithmetic operators taught in school.
Operator | Name | Example | Result |
---|---|---|---|
+ | Addition | $a + $b | Sum of $a and $b |
– | Subtraction | $a – $b | Difference of $a and $b |
* | Multiplication | $a * $b | Product of $a and $b |
/ | Division | $a / $b | Quotient of $a and $b |
% | Modulus | $a % $b | Remainder of $a divided by $b |
** | Exponentiation | $a ** $b | Result of raising $a to the $b’th power |
Assignment Operators and Their Usage
In PHP, the primary assignment operator is ‘=’, which is distinct from the equality operator. It functions by assigning a specific value to a variable.
Example of PHP’s Primary Assignment Operator Usage
<?php
$a = 5;
$b = 7;
$a = $b;
echo $a; // outputs 7
In the given example:
- The value 5 is assigned to the variable $a;
- The value 7 is assigned to the variable $b;
- Subsequently, $a is assigned the value of $b, which is 7;
- Additionally, there are more assignment operators in PHP to explore.
Operator | Example | Long Form | Description |
---|---|---|---|
= | $a = $b | $a = $b | $a gets set to the value of $b |
+= | $a += $b | $a = $a + $b | $a gets set to $a + $b (Addition) |
-= | $a -= $b | $a = $a – $b | $a gets set to $a – $b (Subtraction) |
*= | $a *= $b | $a = $a * $b | $a gets set to $a * $b (Multiplication) |
/= | $a /= $b | $a = $a / $b | $a gets set to $a / $b (Division) |
%= | $a %= $b | $a = $a % $b | $a gets set to $a % $b (Modulus) |
**= | $a **= $b | $a = $a ** $b | $a gets set to $a ** $b (Exponentiation) |
PHP’s Comparison Operators
Comparison operators in PHP are used for evaluating two values based on various criteria.
Operator | Name | Example | Result |
---|---|---|---|
== | Equal | $a == $b | True if values of $a and $b are equal |
=== | Identical | $a === $b | True if both values and data types of $a and $b are equal |
!= | Not equal | $a != $b | True if $a is not equal to $b |
<> | Not equal | $a <> $b | True if $a is not equal to $b |
!== | Not identical | $a !== $b | True if $a is not equal to $b or they are not the same type |
> | Greater than | $a > $b | True if $a is greater than $b |
< | Less than | $a < $b | True if $a is less than $b |
>= | Greater than or equal to | $a >= $b | True if $a is greater than or equal to $b |
<= | Less than or equal to | $a <= $b | True if $a is less than or equal to $b |
<= Less than or equal to $a <= $b True if $a is less than or equal to $b
Note: The operators “!==” and “<>” are functionally identical, serving the same purpose in comparisons.
HP’s Logical Operators and Their Functions
Logical operators in PHP are essential for performing boolean logic operations. They evaluate conditions and return a boolean value (true or false).
Operator | Name | Example | Result |
---|---|---|---|
and | and | $a and $b | True if both $a and $b are true |
&& | and | $a && $b | True if both $a and $b are true |
or | or | $a or $b | True if either $a or $b is true |
|| | or | $a || $b | True if either $a or $b is true |
xor | xor | $a xor $b | True if either $a or $b is true, but not both |
! | not | !$a | True if $a is not true |
Note: The details of the ‘if’ blocks used in these examples will be covered in the next lesson.
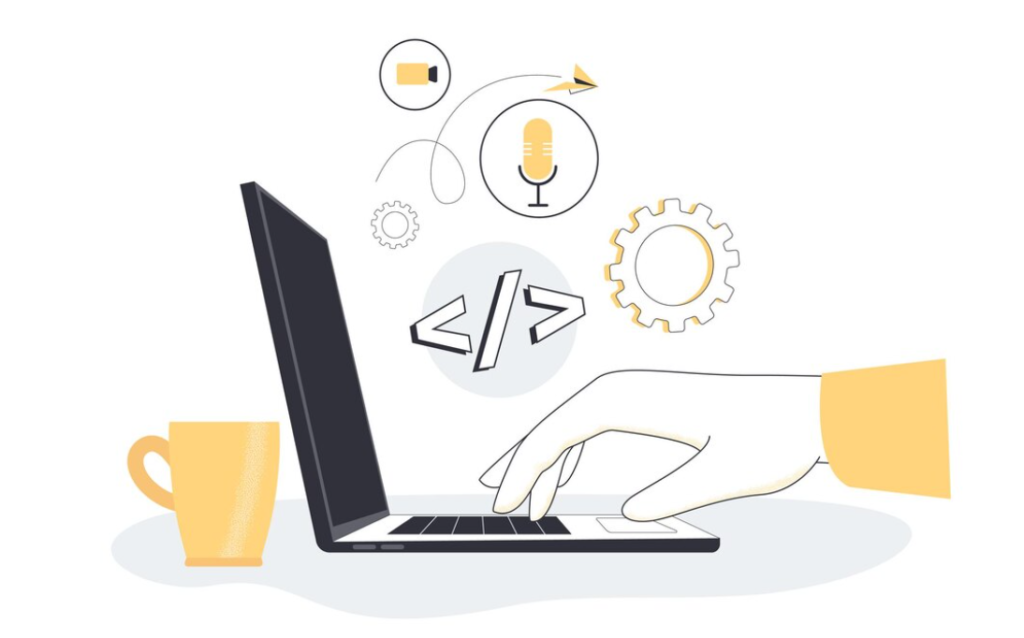
PHP’s Increment/Decrement Operators
PHP provides specific operators for incrementing and decrementing numeric and string values. These operators offer a convenient way to adjust the value of a variable either upwards or downwards.
Key Points:
- These operators are applicable only to strings and numeric data types, including integers and floats;
- Arrays and objects are not influenced by increment/decrement operators;
- While decrementing a null value has no effect, incrementing a null value will result in 1.
Example | Name | Result |
---|---|---|
++$a | Pre-increment | Increments $a by one, then returns $a |
$a++ | Post-increment | Returns $a, then increments $a by one. |
–$a | Pre-decrement | Decrements $a by one, then returns $a |
$a– | Post-decrement | Returns $a, then decrements $a by one. |
PHP String Operators and Their Uses
In PHP, string operators are used to manipulate strings in various ways. These operators are essential for combining, appending, and modifying strings. Below is a table outlining the two primary string operators in PHP and their applications:
Operator | Name | Example | Result |
---|---|---|---|
. | Concatenation | $a . $b | Returns the concatenation of $a and $b |
.= | Concatenation Assignment | $a .= $b | Sets $a to the concatenation of $a . $b |
PHP Array Operators
In PHP, array operators are powerful tools for manipulating and comparing arrays. These operators enable developers to perform a range of operations, such as combining arrays, checking equality, and assessing identicalness. Here’s an table that includes these array operators and their functionalities:
Operator | Name | Example | Result |
---|---|---|---|
+ | Union | $a + $b | Returns the union of $a and $b arrays, preserving keys from the left array ($a). |
+= | Union Assignment | $a += $b | $a gets set to the union of $a and $b, with $a retaining its original keys. |
== | Equal | $a == $b | True if $a and $b have equal key/value pairs, irrespective of order and data types. |
=== | Identical | $a === $b | True if $a and $b have equal key/value pairs in the same order and of the same data type. |
!= | Not equal | $a != $b | True if $a and $b are not equal in their key/value pairs. |
<> | Not equal | $a <> $b | True if $a and $b are not equal, alternative syntax to ‘!=’. |
!== | Not identical | $a !== $b | True if $a and $b have different orders, key/value pairs, or values of different types. |
PHP Spaceship Operator
The Spaceship Operator (<=>), introduced in PHP 7, is a versatile combined comparison operator used for comparing integers, floats, and strings. This operator provides a concise way to perform three-way comparisons between its left and right operands.
Here’s a detailed table explaining the return values of the Spaceship Operator in different scenarios:
Condition | Return Value |
---|---|
Both sides are equal | 0 |
The left side is greater | 1 |
The right side is greater | -1 |
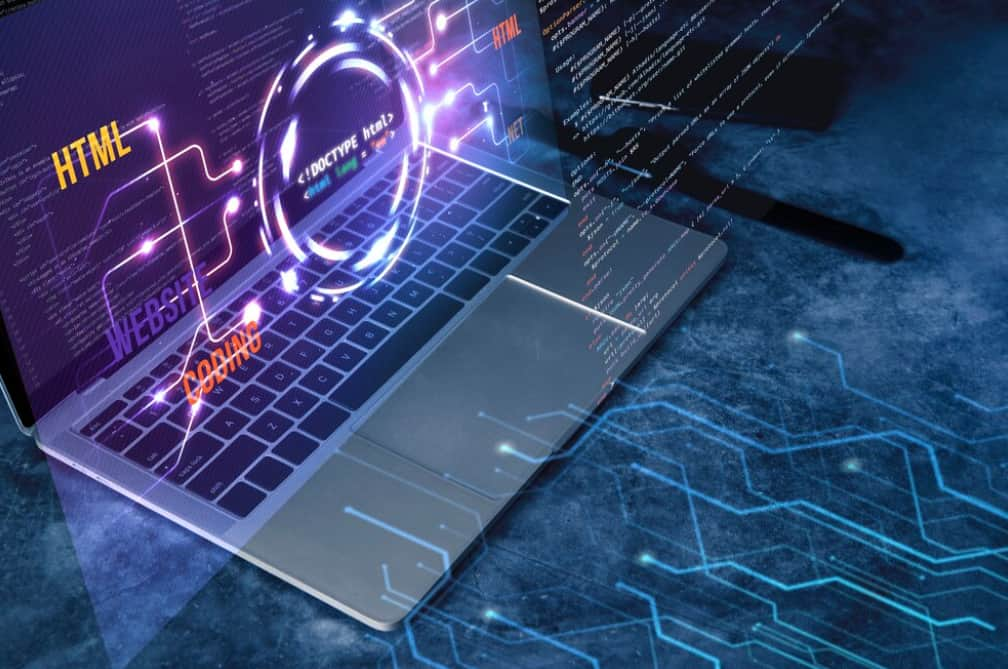
Integer Comparison
<?php
var_dump(1 <=> 1); // outputs 0
var_dump(1 <=> 2); // outputs -1
var_dump(2 <=> 1); // outputs 1
Float Comparison
<?php
var_dump(1.5 <=> 1.5); // outputs 0
var_dump(1.5 <=> 2.5); // outputs -1
var_dump(2.5 <=> 1.5); // outputs 1
String Comparison
<?php
var_dump('a' <=> 'a'); // outputs 0
var_dump('a' <=> 'b'); // outputs -1
var_dump('b' <=> 'a'); // outputs 1
var_dump('eat' <=> 'ear'); // outputs 1
Mixed Comparison
<?php
var_dump(1 <=> 2.5); // outputs -1
var_dump(2.5 <=> 1); // outputs 1
var_dump('1' <=> 1); // outputs 0
var_dump(1 <=> '2'); // outputs -1
var_dump('2' <=> 1); // outputs 1
Comparing a string such as “dog” with an integer like 10 can lead to surprising outcomes.
Conclusion
Operators in PHP play a crucial role in performing actions between variables and values. With a variety of 11 different operator types, PHP offers programmers a vast toolkit for arithmetic, assignment, comparison, and more. Mastering these operators, understanding when and how to use them, can significantly enhance your PHP coding efficiency. It’s also important to stay updated as new operators, such as the Spaceship Operator in PHP 7, are introduced regularly, offering more optimized solutions. Continue learning and experimenting with these operators to get the most out of your PHP development journey.