In the ever-evolving landscape of web development, PHP has firmly established itself as a versatile and powerful scripting language. With its extensive libraries and frameworks, PHP provides developers with the tools to create dynamic and feature-rich web applications. One of the key features that sets PHP apart from other scripting languages is its support for Object-Oriented Programming (OOP), a paradigm that promotes code organization, reusability, and maintainability.
In this comprehensive guide, we will dive deep into the world of PHP OOP static, exploring its various applications, best practices, and potential pitfalls. Whether you’re a seasoned PHP developer looking to enhance your skills or a newcomer eager to grasp the nuances of OOP static, this article will serve as your roadmap to harnessing the full potential of this intriguing aspect of PHP development. Let’s embark on a journey to unlock the power of PHP OOP static and elevate your coding expertise to new heights.
Static Methods – Declaration
Static methods are a fundamental aspect of object-oriented programming, serving as powerful tools in any developer’s arsenal. They allow you to create functions within a class that are not tied to a specific instance of that class. Here’s how you declare static methods and some insights into visibility modifiers:
1. Declaration of Static Methods:
When you want to create a static method in a class, you use the static keyword. Additionally, you can specify a visibility modifier to control the access level to the static method. If you omit the visibility modifier, it defaults to public, but you can choose from public, private, or protected, depending on your needs. The same visibility rules that apply to regular methods are also applied to static methods.
class MyClass {
public static function myStaticMethod() {
echo "Hyvor!";
}
}
2. Visibility Modifiers for Static Methods:
- Public: If you declare a static method as public, it can be accessed from anywhere, both inside and outside the class;
- Private: A private static method can only be accessed within the class that defines it. It’s hidden from the outside world;
- Protected: When you declare a static method as protected, it can be accessed within its defining class and its child classes, facilitating inheritance.
By understanding and utilizing these visibility modifiers, you can precisely control the accessibility of your static methods.
Static Methods – Accessing
Now that you’ve declared your static methods let’s dive into how you can access them, including inheritance considerations and usage within the same class:
1. Accessing Static Methods:
Static methods are called using the class name followed by the Scope Resolution Operator (::). However, this is only possible if the visibility of the static method is set to public.
Example: MyClass::myStaticMethod(); // Accessing a public static method
2. Accessing Static Methods from Other Classes:
You can also call static methods from methods of other classes, but this requires the visibility of the static method to be public. Here’s how you can do it:
class OtherClass {
public function myMethod() {
MyClass::myStaticMethod(); // Visibility of myStaticMethod should be public
}
}
3. Inheritance and Static Methods:
When dealing with inheritance, you have a bit more flexibility regarding the visibility of static methods. To access a static method from child classes of the class where the static method is declared, the visibility can be set to protected. This enables child classes to inherit and use the static method.
Example:
class OtherClass extends MyClass {
public function myMethod() {
MyClass::myStaticMethod(); // Visibility of myStaticMethod should be public or protected
// or
parent::myStaticMethod(); // Using the parent keyword
}
}
4. Parent Keyword Inside Child Classes:
The parent keyword inside a child class refers to its parent class. It is especially useful when calling static methods inherited from the parent class.
Example:
class Hyvor {
protected static function getCompanyName() {
return 'Hyvor, Inc.';
}
}
class HyvorAuth extends Hyvor {
public $companyName;
public function __construct() {
$this->companyName = parent::getCompanyName();
}
}
$hyvorAuth = new HyvorAuth;
echo $hyvorAuth->companyName; // Outputs: Hyvor, Inc.
5. Accessing Static Methods from Non-Static Methods:
Static methods can also be accessed from non-static methods within the same class using the self keyword and the Scope Resolution Operator (::). This can be handy when you want to encapsulate related functionality within a class.
class MyClass {
public static function myStaticMethod() {
echo "Hyvor!";
}
public function myMethod() {
self::myStaticMethod(); // Calling a static method from a non-static method
}
}
By mastering these concepts, you can harness the power of static methods, control their accessibility, and utilize them effectively in your PHP code.
Static Methods – An In-Depth Exploration
In the realm of PHP programming, understanding the concept of static methods is pivotal. These methods provide a unique functionality that allows you to manipulate class data without the need to create instances of the class. Let’s delve into this topic with a comprehensive example and insights:
PHP Static Methods Example
class MyClass {
public static function callMe() {
echo "Hyvor!";
}
public function __construct() {
self::callMe();
}
}
MyClass::callMe();
echo "<br>";
new MyClass();
Class with Both Static and Non-Static Methods:
- The example showcases a class, MyClass, which contains both static and non-static methods. This combination allows for versatile use within your codebase;
- Static methods provide a convenient way to access specific functions within a class without creating an instance of the class.
Constructor with Static Method Call:
- In the constructor of MyClass, we call the static method callMe() using self::callMe(). This demonstrates that static methods can be invoked within class constructors;
- This approach can be particularly useful when you want certain actions to occur automatically when an object is instantiated.
Usage and Output:
- By calling MyClass::callMe(), you can directly invoke the static method and output “Hyvor!” to the screen;
- The instantiation of new MyClass() also triggers the constructor, effectively calling the static method and providing a seamless user experience.
Static Properties – A Closer Look
Static properties are a fundamental component of PHP classes, allowing for the creation and manipulation of shared data across instances. Here, we delve into declaring, accessing, and altering static properties:
Static Properties – Declaring
class MyClass {
public static $myStaticProperty = 'Hyvor';
}
Declaration of Static Properties:
- In this example, we declare a static property named $myStaticProperty within the MyClass class;
- Static properties are initialized once for the entire class and can be accessed and modified without creating class instances.
Static Properties – Accessing
Accessing static properties is straightforward, following the same principles as invoking static methods. The visibility rules established for properties also apply to static ones.
class MyClass {
public static $name = 'Hyvor';
public function __construct() {
echo 'We are ' . self::$name;
}
}
echo MyClass::$name . '<br>';
MyClass::$name = 'Hyvor Developer';
new MyClass();
- Within the MyClass class, we define a static property named $name;
- In the constructor, we showcase how to access and display the static property value using self::$name. This demonstrates the seamless integration of static properties within class methods;
- Modifying Static Properties: You can dynamically change the value of a static property, just like you would with regular variables. In this example, we alter $name from ‘Hyvor’ to ‘Hyvor Developer’;
- Creating Class Instances: Finally, we create a new instance of MyClass. This action invokes the constructor method, allowing us to access and display the updated static property value.
Exploring the Benefits of Static Methods and Properties in PHP
Static methods and properties are essential elements in the world of PHP programming, offering a unique set of advantages and capabilities for developers. In this comprehensive guide, we’ll delve into the reasons behind the widespread use of static methods and properties, their practical applications, and how they can enhance the organization and efficiency of your PHP code.
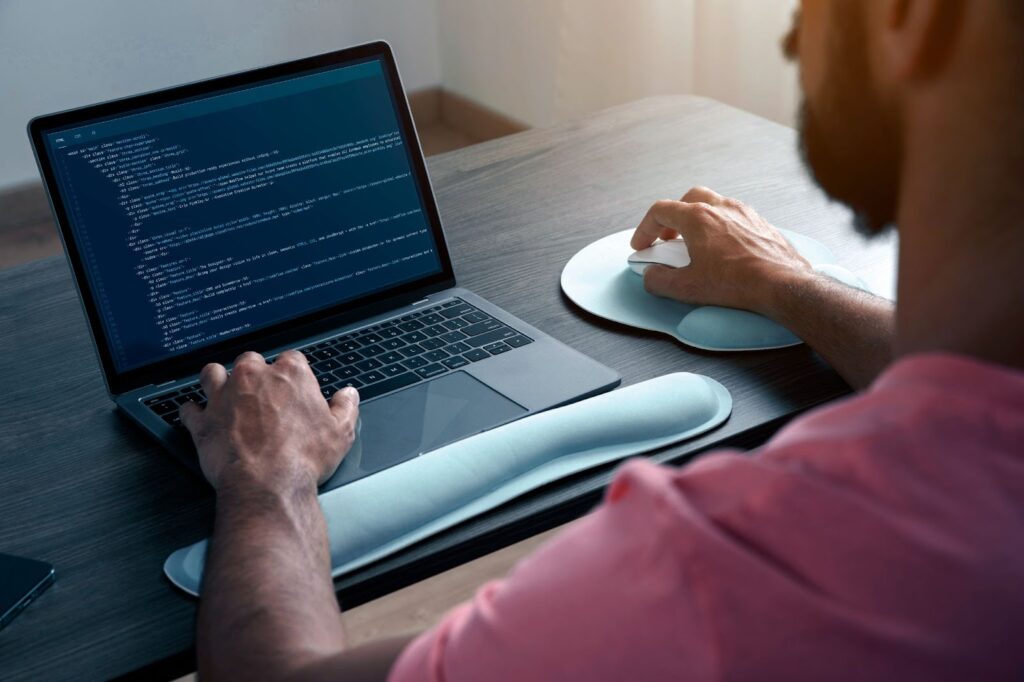
Why Utilize Static Methods and Properties?
Static methods and properties find their niche in PHP primarily to provide additional support for classes. While it may raise the question of why not use regular functions instead, embracing the Object-Oriented Programming (OOP) paradigm unlocks a multitude of benefits. Here’s why you should consider incorporating static methods and properties into your PHP code:
- Organization and Structure:
- Static methods allow you to group related functions within a class, promoting a more organized codebase;
- They provide a clear and logical structure, making it easier to locate and manage specific functionalities.
- Code Reusability:
- Static methods encourage the reusability of code snippets, as they can be accessed across different parts of your application without the need to recreate the same function multiple times.
- Autoloading and Autoloading Classes:
- One of the standout features of OOP is autoloading, a practice that automatically includes class files when needed;
- By encapsulating helper or utility functions within a class, you enable seamless
- Improved Code Documentation:
- Utilizing static methods within a class aligns with the PHP DocBlocks standard for comments, facilitating clear and informative code documentation;
- Well-documented code becomes more manageable and maintainable, especially when collaborating with other developers.
Example Usage of Static Methods in a Class
Now that we’ve highlighted the advantages, let’s dive into a practical example to illustrate the use of static methods within a class:
class User {
public $firstname;
public $lastname;
/**
* @param string $firstname - Firstname of the user
* @param string $lastname - Lastname of the user
*/
public function __construct($firstname, $lastname) {
$this->firstname = self::filterName($firstname);
$this->lastname = self::filterName($lastname);
}
/**
* @param string $name - name to filter
* @return string - filtered name
*/
static function filterName($name) {
// Remove surrounding whitespaces
$name = trim($name);
// Remove non-latin and non-number characters
$name = preg_replace('/[^a-zA-Z0-9]/', '', $name);
return $name;
}
}
A Closer Look at the Example:
We’ve created a class called “User” to handle user data, which includes first and last names.
Both first names and last names require filtering. Since the filtering method, “filterName(),” does not need access to the instance variables, it can be made a static method.
Take note of the informative comments. These are referred to as PHP DocBlocks, a widely adopted standard in PHP development for writing detailed and well-organized comments. Adhering to this standard enhances code readability and documentation. Also, discover the magic of Live Search, where instant results meet your queries, making online exploration a breeze. Dive in now!
Conclusion
In conclusion, exploring the concept of PHP Object-Oriented Programming (OOP) with a focus on static methods and properties has revealed the versatility and power of this programming paradigm. Static methods and properties allow developers to create reusable code that can be accessed without the need for object instantiation. This not only enhances code organization but also improves code efficiency by reducing unnecessary object creation.
By embracing PHP’s OOP capabilities, developers can build more maintainable, scalable, and modular applications. Static methods and properties serve as valuable tools in the toolkit of PHP developers, enabling them to design elegant and efficient solutions for a wide range of programming challenges.