In the realm of PHP’s Object-Oriented Programming (OOP), the term “methods” refers to the functions nested within classes. Although these methods have a similar declaration structure and behavior to standard functions, they are unique in their usage and purpose within the context of the class. Here, we will dive into the operations and applications of these vital PHP components, offering a clear and concise understanding of this programming paradigm.
Unfolding the Function of Methods
To better understand what a method is, let’s first review the primary function’s role:
- Declaration: Defining the function initially is a vital step that sets the stage for its later use. The function’s syntax, parameters, and purpose are outlined during this phase;
- Invocation: After declaring the function, it’s time to call it into action. Although optional, we can send arguments into the function as needed to manipulate the data or perform certain processes;
- Processing: Within the function, various computations or operations are carried out. These processes can endure data handling, mathematical computations, or any other actions necessary to accomplish the function’s defined purpose;
- Return: Although this is optional, a function often concludes by returning something. The return value could be the result of a computation, a manipulated string, or any other data processed within the function.
When it comes to methods in PHP, they work in a similar way but within a class. Each method encapsulated in a class can directly access and manipulate the class’s properties. They’re like private, inner workers, acting to achieve specific tasks related to the class, making code more organized and object-oriented.
Illustrating Method Declaration
To visualize how this works, let’s create a method within an exemplary class titled “SampleClass”. This method will simply emit a given string.
<?php
class SampleClass {
public function display($input) {
echo $input;
}
}
In the class “SampleClass”, we’ve declared a public function named “display”. This method accepts an argument, $input. When this method is summoned, it will echo, or output, the $input string.
Making a Method Call
Have a look at the following code snippet.
$demoInstance = new DemoClass();
$demoInstance->display('Greetings, World!');
And just like that, you’ve executed your first method call! But what exactly happened?
Output: Greetings, World!
Here’s a detailed breakdown:
- We begin by generating an object, $demoInstance, using the DemoClass class. In PHP, a new object of a class is created using the new keyword, followed by the name of the class;
- Following this, we invoke the display method using the -> notation, also known as the object operator, and enveloping parentheses. The object operator is used to access methods and properties of an object in PHP;
- Inside the parentheses, we include the arguments, which, in our case, is the string ‘Greetings, World!’.
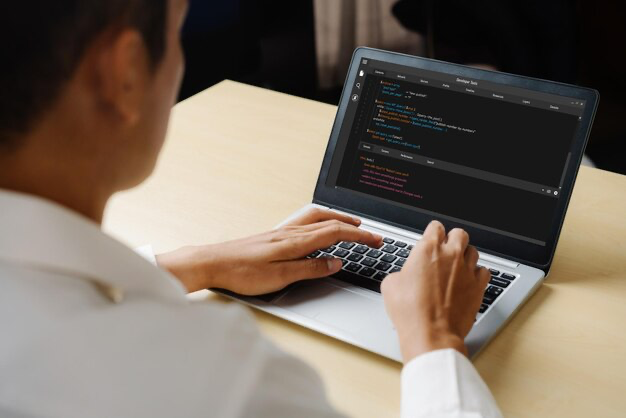
Property Modification Through Methods: A Real-World Example
In Object-Oriented Programming with PHP, methods come with the power to alter the properties of a class. A prime example of this can be seen in a hypothetical Building class, where we can modify the mainColor attribute of the class using a method.
Consider the Building class with a single property $mainColor, which defaults to ‘grey’. We’ll then implement a method to change this mainColor.
class Building {
public $mainColor = 'grey';
public function repaint($newColor) {
$this -> mainColor = $newColor;
}
}
- The Building class has a public property mainColor, initially set to ‘grey’;
- The repaint method allows us to change mainColor to any color we prefer.
Next, let’s see how we can use an instance of this class:
// instance creation$myBuilding = new Building();// display initial colorecho $myBuilding -> mainColor;// repaint the building$myBuilding -> repaint('blue');// display updated colorecho $myBuilding -> mainColor;
Output: grey blue
In this example:
- We first create an instance of the Building class, $myBuilding;
- To check the initial color, we echo $myBuilding -> mainColor, and it outputs ‘grey’;
- To change the color, we call the repaint method on $myBuilding, passing ‘blue’ as the argument. This changes the mainColor of $myBuilding to ‘blue’;
- Finally, when we echo $myBuilding -> mainColor again, it now outputs ‘blue’.
And there you have it – properties of class instances (objects) can be effortlessly modified using class methods. Grasping this concept is fundamental to harnessing the power of object-oriented programming with PHP. Essentially, methods serve as gateways into classes, allowing us to interact with and manipulate the class properties. Armed with this knowledge, you’re now one step closer to becoming an efficient PHP programmer!
Constructor and Destructor in PHP
In the realm of PHP’s Object-Oriented Programming, it’s imperative to acquaint oneself with the concepts of constructors and destructors. These specialized methods play a pivotal role in the lifecycle of a class. A constructor, denoted by the “__construct” method, is invoked automatically upon the creation of a new object from a class. It is often employed to initialize object properties and perform essential setup tasks. Conversely, the destructor, designated as “__destruct,” is called when an object is no longer in use, allowing for cleanup operations such as closing database connections or releasing resources. Understanding the synergy between methods, including constructors and destructors, furthers your prowess in harnessing PHP’s OOP capabilities effectively.
In Conclusion
In the grand scheme of PHP’s Object-Oriented Programming, understanding the role of methods within classes is a game-changer. These special class functions enable you to effectively manipulate, access, and customize an object’s properties and behaviors. Mastering methods will significantly streamline your coding processes, resulting in more efficient and cleaner scripts. The deep dive into PHP’s methods and their applications offered in this article provides valuable knowledge for any coder, from beginners to seasoned PHP programmers.