In the dynamic world of web development, PHP remains a stalwart, driving countless websites and applications. Its versatility and extensibility make it a favorite among developers, enabling them to create robust and scalable codebases. One fundamental concept that plays a pivotal role in achieving these goals is Object-Oriented Programming (OOP). Within the realm of OOP, inheritance stands as a cornerstone, offering a powerful mechanism for code reusability, organization, and flexibility.
In this article, we embark on a journey into the realm of PHP OOP inheritance. We’ll unravel the intricacies of this concept, understand its benefits, and explore how it can significantly enhance the way we architect and maintain PHP applications. Whether you’re a seasoned PHP developer looking to deepen your knowledge or a newcomer eager to grasp the essentials, this exploration of PHP OOP inheritance promises to illuminate the path to more efficient and elegant code. So, let’s dive in and uncover the secrets of this essential PHP programming paradigm.
Unlocking the Power of Inheritance in Object-Oriented Programming
In the fascinating realm of Object-Oriented Programming (OOP), inheritance reigns supreme as a foundational concept. Imagine it as the DNA that shapes the classes within this coding ecosystem. At its core, inheritance allows a class to inherit characteristics and behaviors from another, akin to a generational hand-me-down. Let’s delve deeper into this concept and unveil its intriguing facets:
1. The Parent-Child Relationship
Inheritance in OOP is like a family tree, where one class begets another. The class that derives from another is affectionately known as the “child” class, while the source class is the “parent” class. Occasionally, these are referred to as the “sub” class and “super” class, respectively. It’s akin to a generational transfer of attributes and methods, where the child inherits the legacy of the parent.
2. Extending Horizons
Picture the child class as a vine reaching out to new horizons. It extends or expands upon the functionalities of the parent class, breathing fresh life into the code. The child class can inherit all the public and protected properties and methods from the parent class, thus gaining a strong foundation. Simultaneously, it has the freedom to introduce its own unique properties and methods.
3. Inheritance Cascade
In the intricate world of OOP, the lineage of inheritance doesn’t end with a single child. A child class can become a parent itself, propagating its traits to its own offspring. This creates a cascading effect, enabling complex hierarchies of classes and relationships.
4. The Precursor of Abstraction and Interfaces
As you advance in OOP, you’ll discover that concepts like abstraction and interfaces have their roots deeply entwined with inheritance. These concepts enable you to define structures and enforce contract-like behaviors in your code, making it more organized and maintainable.
5. PHP’s Inheritance Syntax
In PHP, the “extends” keyword is the magic wand for declaring an inherited class. This keyword acts as a bridge, connecting the parent and child classes in a harmonious coding ballet.
Read about the power of PHP’s static function, and discover how it enhances your coding prowess. Dive into the world of efficiency and versatility today!
Let’s Observe PHP Inheritance in Action
Consider a real-world analogy: animals. Dogs and cats, like classes, share common behaviors, yet each has its unique quirks. In this analogy, let’s create a “Person” class as the parent and a “Tom” class as the child.
PHP Inheritance Example:
class Person {
public $name;
public $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
public function introduce() {
echo "My name is {$this->name}. My age is {$this->age}";
}
}
/* Tom is inherited from Person */
class Tom extends Person {
# __construct() is inherited
# introduce() is inherited
public function sayHello() {
echo "Hello, World <br>";
}
}
$tom = new Tom('Tom', 29);
$tom->sayHello();
$tom->introduce();
Protected Visibility for Inheritance: Expanding Your Knowledge
In our previous discussions on visibility, we’ve covered both public and private visibility with several examples. However, one vital aspect that warrants a more thorough exploration is “protected visibility.” In the realm of object-oriented programming, protected visibility plays a crucial role, primarily in the context of inheritance. Let’s delve into the intricacies of protected visibility, its applications, and how it can be harnessed effectively.
Understanding the Purpose of Protected Visibility
Protected visibility serves as a means to encapsulate class members (properties and methods) in such a way that they are accessible not only within the class where they are defined but also within the classes that inherit from the parent class. This provides a powerful mechanism for controlling access to certain class elements, ensuring that they are used only in designated contexts. When you declare a property or method as protected, it becomes available for use in two distinct scenarios:
- Within the Class Where It is Defined: Members with protected visibility can be freely accessed and manipulated within the class where they are originally defined. This ensures that the class itself can utilize these elements as needed for its internal functionality;
- Within the Classes Which Inherit the Defined Class: Perhaps the most critical aspect of protected visibility lies in its impact on inheritance. When a class inherits from another class that has protected members, the child class gains access to those protected members. This paves the way for building upon the functionality of the parent class while maintaining control over the visibility of inherited elements.
Practical Example: PHP Protected Visibility
Let’s explore a practical example in PHP to illustrate the concept of protected visibility:
class ParentClass {
protected $protectedProperty = 'Protected';
private $privateProperty = 'Private';
protected function protectedMethod() {
echo $this->protectedProperty;
}
private function privateMethod() {
// This method cannot be called from Child
}
}
class Child extends ParentClass {
public function doSomething() {
$this->protectedMethod(); // This is valid
// $this->privateMethod(); // This is invalid, as private methods are not accessible
}
}
$child = new Child();
$child->doSomething();
In this example, we can see how the protected property $protectedProperty and the protected method protectedMethod() are accessible within the Child class, as it inherits from ParentClass. Meanwhile, the private property and method remain hidden from the child class.
Overriding Inherited Methods: Customizing Functionality
Another critical aspect of inheritance in object-oriented programming is the ability to override inherited methods. This allows child classes to customize or extend the behavior of their parent classes. Let’s explore the concept of method overriding in PHP and how it can be employed effectively to tailor the behavior of classes.
Method Overriding: A Powerful Inheritance Feature
Method overriding is the process of redefining a method in a child class with the same name as a method in the parent class. This action allows the child class to provide its own implementation of the method, effectively replacing or extending the behavior inherited from the parent class. Here’s a practical PHP example to illustrate method overriding:
class Person {
public $name;
public $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
public function introduce() {
echo "My name is {$this->name}. My age is {$this->age}";
}
}
class Tom extends Person {
public $school;
public function __construct($name, $age, $school) {
parent::__construct($name, $age); // Call the parent class constructor
$this->school = $school;
}
public function introduce() {
echo "My name is {$this->name}. My age is {$this->age}. My school is {$this->school}";
}
}
$tom = new Tom('Tom', 29, 'Foothill School');
$tom->introduce();
In this example, the Tom class overrides both the __construct() and introduce() methods inherited from the Person class. This customization allows Tom to provide specific information about his school, in addition to the general introduction inherited from Person.
Exploring the Art of Calling Parent’s Methods in PHP
In the world of object-oriented programming in PHP, one of the essential skills is knowing how to call parent methods from a child class. In this fascinating journey, we’ll delve into the ins and outs of this concept and uncover the power of the Scope Resolution Operator (::). Brace yourself for an adventure that will unlock a whole new realm of coding possibilities.
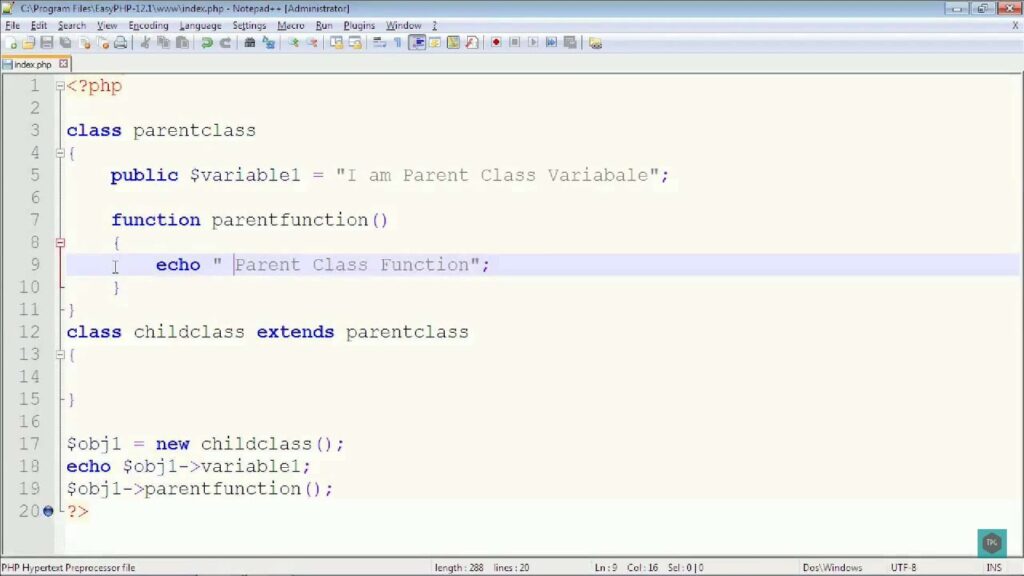
The Mighty Scope Resolution Operator (::)
The Scope Resolution Operator, also known as ::, is a unique syntactical gem in PHP’s object-oriented paradigm. It serves as a bridge connecting child and parent classes, allowing you to access parent methods with elegance and precision. While we’ll explore its magic further, let’s first highlight some crucial points:
- The Scope Resolution Operator facilitates interactions between child and parent classes;
- It is a key player in the realm of object-oriented programming in PHP;
- Intriguingly, the full potential of :: is yet to be unveiled, but stay tuned as we unravel its mysteries in upcoming chapters.
Embracing the Change: Calling Parent Constructors
Picture this scenario: you have a child class, and you want to call the constructor of its parent class. Fear not, for the solution is simple and elegant:
parent::__construct();
Here’s a breakdown of what’s happening:
- parent:: signifies that we are tapping into the parent class;
- __construct() is the constructor method we’re calling.
Let’s illustrate this with a real-world example:
PHP Calling Parent’s Methods
class Person {
public $name;
public $age;
public function __construct($name, $age) {
$this->name = $name;
$this->age = $age;
}
public function introduce() {
echo "My name is {$this->name}. My age is {$this->age}";
}
}
class Tom extends Person {
public $school;
public function __construct($name, $age, $school) {
parent::__construct($name, $age); // Call the parent's constructor
$this->school = $school;
}
public function introduce() {
echo "My name is {$this->name}. My age is {$this->age}. My school is {$this->school}";
}
}
$tom = new Tom('Tom', 29, 'Foothill School');
$tom->introduce();
Now, when we create an instance of the Tom class, it seamlessly inherits the properties and methods from Person while adding its unique touch.
Navigating the Challenge of Overriding
In the realm of PHP inheritance, overriding parent methods is a common practice. However, a challenge arises when you need to differentiate between the child’s method and the parent’s method. In such cases, the parent::methodName construct becomes your trusty companion.
Consider this scenario:
When you override a parent’s method, you cannot use $this->methodName to refer to the parent’s method, as it now points to the child class’s method. Instead, rely on parent::methodName to access the parent’s version.
Harnessing the Power of the Final Keyword
The final keyword adds another layer of control to your PHP classes, preventing inheritance and method overriding. Let’s explore its two primary uses:
Preventing Class Inheritance: Declare a class as final, and it becomes impervious to any attempts at inheritance.
final class NonParent {
// Class code
}
// This will throw an error
class Child extends NonParent {...}
Preventing Method Overriding: Mark a method as final, and no child class can override it.
php
Copy code
class ParentClass {
final public function myMethod() {
// Method code
}
}
class Child extends ParentClass {
// Fatal Error: Overriding final methods
public function myMethod() {
// Attempting to override a final method
}
}
The final keyword acts as a guard, protecting your classes and methods from unwanted alterations, ensuring code stability.
Conclusion
In conclusion, PHP Object-Oriented Programming (OOP) Inheritance is a powerful and fundamental concept that allows developers to create efficient and organized code. By building upon existing classes and reusing code, inheritance promotes code reusability, maintainability, and scalability. Through this article, we have explored the principles of inheritance in PHP, including extending classes, overriding methods, and accessing parent class members. We have also discussed the benefits and best practices of using inheritance in PHP OOP.
Inheritance not only simplifies code development but also enhances its flexibility, allowing for the creation of complex and feature-rich applications with ease. It encourages a modular and structured approach to programming, making it easier to manage and extend your codebase as projects grow.