Alt: hard drive near the laptop on the table under the blue and red light and coding on the fond
The PHP list() function is a valuable tool for developers that enables the assignment of array elements to variables in a direct and readable manner. As of PHP 7.1, this function has been complemented by shorthand array destructuring, which offers a more concise syntax. In this article, we’ll explore both the list() function and the shorthand array destructuring syntax, providing insights into their usage and advantages in PHP development.
While PHP Array Shorthand is a powerful feature for handling arrays efficiently, it can also be applied in various ways to enhance your skills in PHP, as demonstrated in our PHP regular expressions tutorial.
PHP Array Data Handling with list() Function
When dealing with user profiles in PHP, information is often stored in indexed arrays. Take for instance the following snippet:
$profile = ['James', 25, 'MIT'];
In this array, $profile, each element corresponds to a user’s details such as name, age, and university. Traditionally, to access each piece of information, you might assign each element to a separate variable like this:
$name = $profile[0];
$age = $profile[1];
$uni = $profile[2].
This approach, while straightforward, becomes cumbersome and verbose as the number of variables increases. Imagine having to write a separate line of code for each element if your $profile array contained 5 or more elements. This is not an efficient way to handle multiple data points.
PHP list() Construct for Array Destructuring
The list() construct in PHP is a practical tool for array destructuring, which allows assigning multiple array elements to separate variables in one go.
Here’s how it works:
list($name, $age, $uni) = $profile;
// $name is 'James'
// $age is 25
// $uni is 'MIT'
list() is straightforward: give it an array, and it assigns the values to variables you define.
But watch out for missing elements. If your array doesn’t have a value for a variable, like if $profile doesn’t have a third item, PHP will generate a notice. So, before using list(), it’s essential to ensure your array has all the elements you’re going to assign.
Simplified Variable Assignment with PHP’s Shorthand Syntax
PHP’s shorthand for the list() function is represented by [], which can also be seen as an array construct.
Here's how you use it:
[$name, $age, $uni] = $
Starting from PHP 7.1, when you place [] on the left side of an assignment operator (=), PHP treats it as shorthand for list(). This update was intended to simplify the way PHP developers assign variables from arrays. However, this new syntax can be mistaken for the array creation syntax, which also uses []. Despite this potential for confusion, it’s a matter of personal or project preference which form to use. For some, the shorthand [] is a preferred choice due to its brevity and modern look.
Unpacking Keys from PHP Associative Arrays
Unpacking or ‘destructuring’ associative arrays in PHP allows you to assign array elements to named variables based on the keys of the array.
Here’s how it’s done:
$profile = [
'name' => 'James',
'age' => 25,
'university' => 'MIT'
];
['name' => $name, 'age' => $age, 'university' => $uni] = $profile;
echo $name; // Outputs 'James'
In this method, each key-value pair is assigned to its corresponding variable. It’s important to check that all keys exist in the array; otherwise, PHP will raise a notice for undefined keys. This approach is particularly handy when you’re handling data retrieved from a database.
For example:
$result = $mysqli->query('SELECT name, email, password FROM users WHERE id = 1 LIMIT 1');
$result = $result->fetch_assoc();
['name' => $name, 'email' => $email, 'password' => $pwd] = $result;
echo $name;
echo $email;
echo $pwd;
Using this technique ensures your variables are neatly assigned to the values from the database without manually indexing each one.
Nested Destructuring for Multi-Dimensional Arrays in PHP
Nested or multi-dimensional arrays are a common occurrence in programming, especially when working with JSON-based APIs that often return nested data structures. PHP supports nested destructuring, which is a handy feature for dealing with these types of arrays.
Consider an example of data from a “Soap Shop”:
<?php
$soapShop = [
'meta' => [
'opened' => true,
'soap-types' => ['Glycerin', 'Transparent', 'Liquid']
],
'isAvailableInStore' => [
'Glycerin' => true,
'Transparent' => true,
'Liquid' => false
]
];
To extract this data into variables, nested destructuring can be used:
[
'meta' => $meta,
'isAvailableInStore' => [
'Glycerin' => $glycerinSoapAvailable,
'Transparent' => $transparentSoapAvailable,
'Liquid' => $liquidSoapAvailable
]
] = $soapShop;
This code does the following:
- Extracts the ‘meta’ key’s value into the $meta variable, which now contains an array with keys ‘opened’ and ‘soap-types’;
- For the ‘isAvailableInStore’ key, rather than assigning its value to a single variable, it further destructures its value into separate variables, demonstrating nested destructuring.
Nested destructuring is a powerful feature in PHP that simplifies the handling of complex, multi-dimensional arrays.
Enhancing Loops with Array Destructuring in PHP
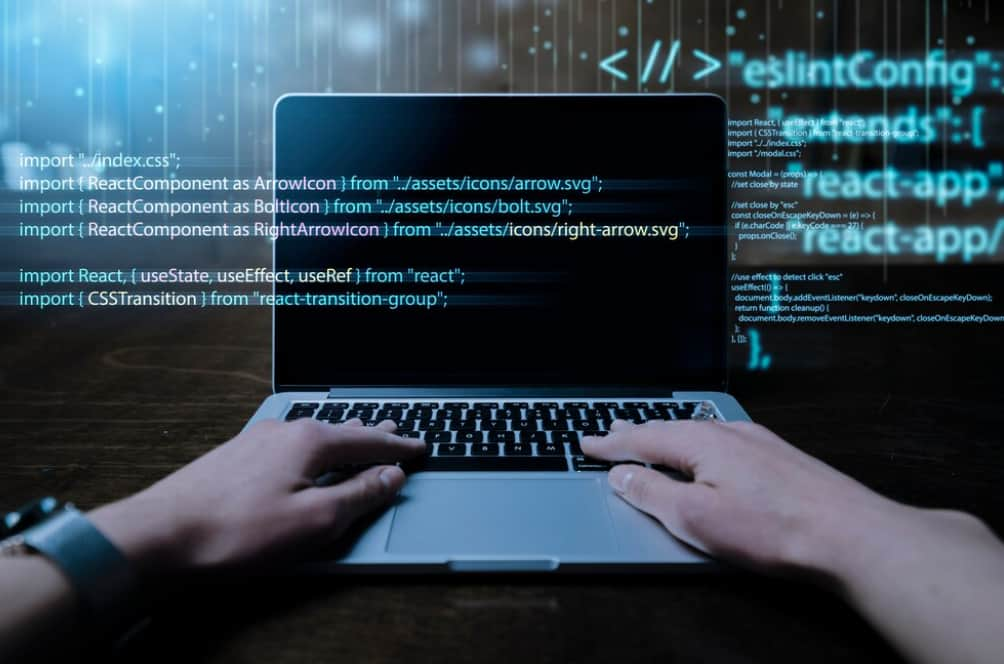
Array destructuring in PHP is a highly effective technique, particularly when iterating over arrays.
Consider the following example:
<?php
$people = [
[
'name' => 'John',
'age' => 20
],
[
'name' => 'Callum',
'age' => 17
]
];
// the old way
/*
foreach ($people as $person) {
echo $person['name'];
echo $people['age'];
}
*/
foreach ($people as ['name' => $name, 'age' => $age]) {
echo $name;
echo $age;
}
In this snippet, array destructuring within the foreach loop allows direct assignment of the ‘name’ and ‘age’ values to the $name and $age variables, respectively.
Here’s another instance of array destructuring with an array of indexed arrays:
<?php
$array = [
[1,2,3],
[4,2,5],
[2,6,4]
];
foreach ($array as [$a, $b, $c]) {
echo $a $b $c;
}
Array destructuring, whether using list() or its shorthand, is particularly useful in loops and can also be effectively combined with PHP functions that return arrays, such as parse_url, pathinfo, or custom functions. This feature not only simplifies code but also makes it more readable and maintainable.
Conclusion
PHP array shorthand and the process of array destructuring can optimize your coding experience, making scripts lighter and more readable. Whether you’re handling simple indexed arrays or grappling with structured associative and nested arrays, PHP’s list() and its shorthand [] provide a comprehensive solution. Additionally, their utility in loops and when used in conjunction with functions that return arrays make them a potent tool in a programmer’s arsenal. Understanding these aspects and integrating them into your regular coding practice can significantly enhance your PHP scripting efficiency, offering faster and more intuitive solutions to complex coding scenarios.